Discord
Discord is the easiest way to talk over voice, video, and text. With this integration, you can easily use Discord API.
Official Websitehttps://discord.js.org/#/
Tagsmessagingbot
JavaScript
Python
NodeJS packagehttps://www.npmjs.com/package/discord.js
Version14.14.1
Source Codehttps://github.com/discordjs/discord.js
Comming soon
We are releasing new Python features every week
Credential configuration
The token
field corresponds to the token of the bot you want to use.
If you don't have a bot, you can create one and get the token following these steps.
For more information about Discord intents, visit this page.
In the extra options field, you can include most of the parameters found here.
Here is an example of a filled credential configuration form in YepCode:
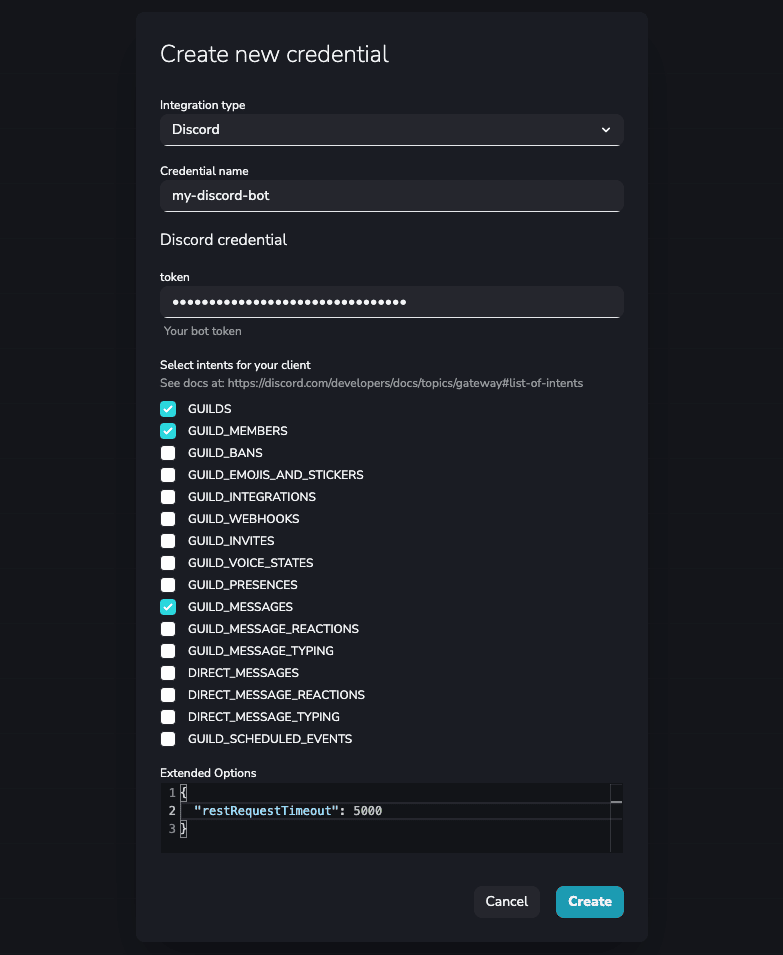
Discord Snippets available in editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const discordClient = yepcode.integration.discord("credential-slug");
New integration from plain authentication data
const { Client, Intents } = require("discord.js");
// You can use the intents you want, or an empty list
const discordClient = new Client({ intents: [Intents.FLAGS.GUILDS] });
discordClient.login("token")
tip
Learn about discord intents here: https://discord.com/developers/docs/topics/gateway#gateway-intents
Close Connection
Close connection
discordClient.destroy()
Get Servers which Bot is in
Get servers which bot is in
discordClient.guilds.fetch({ limit: 10 }).then(servers => {
servers.forEach(server => console.log(`Found server with id: ${server.id}`))
})
Get Server
Get server
const server = await discordClient.guilds.fetch("guildId");
console.log(server)
Get Server Channels
Get server channels
const server = await discordClient.guilds.fetch("guildId");
const channels = await server.channels.fetch();
channels.forEach((channel) => {
console.log(`Found channel: id: ${channel.id} - name: ${channel.name} - type: ${channel.type}`);
});
Get Channel Messages
Get channel messages
const channel = await discordClient.channels.fetch("channelId");
channel.messages.fetch({ limit: 10 }).then((messages) => {
messages.forEach((message) => {
console.log(`Found message with content: ${message.content}`);
});
});
Create a Command
Create a command
const command = await discordClient.application.commands
.create({
name: "command name",
description: "command description",
});
console.log(`Created command with id ${command.id}`)
Delete a Command
Delete a command
discordClient.application.commands.delete(command-id)
.then(() => console.log("Deleted command"))
.catch(console.error);
Comming soon
We are releasing new Python features every week