Github
Github is the site where the world builds software.
Official Websitehttps://github.com/
Tagsrepositorycodesourcegitgithub
JavaScript
Python
Documentationhttps://github.com/octokit/octokit.js#readme
NodeJS packagehttps://www.npmjs.com/package/octokit
Version2.0.14
Source Codehttps://github.com/octokit/octokit.js
Comming soon
We are releasing new Python features every week
Credential configuration
To configure this credential, you'll need a personal access token. If you don't have one yet create it here.
In the extra options field, you can provide most of the parameters found here.
Here is an example of a filled credential configuration form in YepCode:
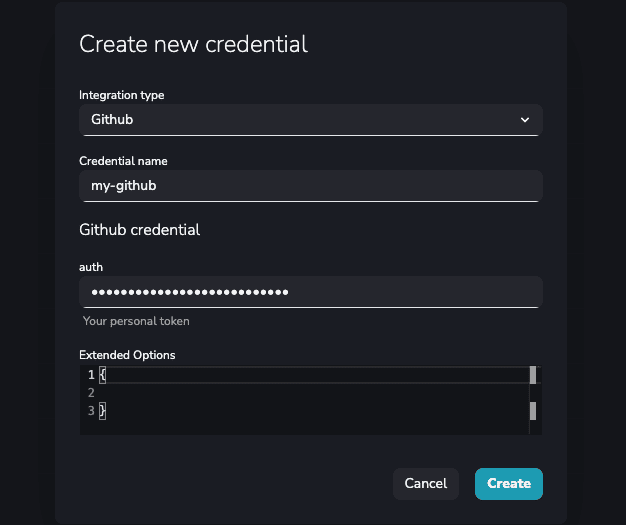
Github Snippets available in editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const githubClient = yepcode.integration.github('credential-slug')
New integration from plain authentication data
const { Octokit } = require("octokit");
const githubClient = new Octokit({ auth: 'your-personal-token' });
Create a Repository
Create repository
const response = await githubClient.rest.repos
.createForAuthenticatedUser({
name: 'your-new-repository-name',
description: `Some description`,
private: true,
auto_init: true
// other props
})
console.log(`Created repository ${response.data.full_name}`);
Delete a Repository
Delete repository
const response = await githubClient.rest.repos
.delete({
owner: 'repository-owner',
repo: 'repository-name',
})
console.log("Deleted repository");
Get a File from Repository
Get file from repository
const response = await githubClient.rest.repos
.getContent({
mediaType: {
format: "raw",
},
owner: 'repository-owner',
repo: 'repository-name',
path: 'path-to-file',
})
console.log(response.data);
Get Commits from Repository
Get commits from repository
const response = await githubClient.rest.repos
.listCommits({
owner: 'repository-owner',
repo: 'repository-name',
per_page: page-size,
page: page-number,
})
const commits = response.data;
commits.forEach((commit) => console.log(`Found commit! ${commit.commit.message}`));
Get Issues from Repository
Get issues from repository
const response = await githubClient.rest.issues
.listForRepo({
owner: 'repository-owner',
repo: 'repository-name',
per_page: page-size,
page: page-number,
state: "open",
})
const issues = response.data;
issues.forEach((issue) => console.log(`Found issue! ${issue.title}`));
Create Issue in a Repository
Create issue in a repository
const response = await githubClient.rest.issues
.create({
owner: 'repository-owner',
repo: 'repository-name',
title: 'issue-title',
body: 'issue-body',
})
console.log(`Created issue! See it here: ${response.data.html_url}`);
Use GraphQL
Use GraphQL
const response = await githubClient
.graphql(
`
query theQuery($owner: String!, $repo: String!) {
theQuery(owner: $owner, name: $repo) {
// the query content
}
}
`,
{
owner: 'repository-owner',
repo: 'repository-name',
}
)
Comming soon
We are releasing new Python features every week