Supabase
Supabase is an open source Firebase alternative. Start your project with a Postgres database, Authentication, instant APIs, Edge Functions, Realtime subscriptions, and Storage.
Official Websitehttps://supabase.com/
TagssupabasefirebasedatabaseSQL
JavaScript
Python
Documentationhttps://supabase.com/docs/reference/javascript
NodeJS packagehttps://www.npmjs.com/package/@supabase/supabase-js
Version2.39.3
Source Codehttps://github.com/supabase/supabase-js
Comming soon
We are releasing new Python features every week
Credential configuration
To configure this credential, you only need the unique project URL (supabaseUrl) and the API key (supabaseUrl). Both can be found navigating to Settings > API in your Supabase account.
Here is an example of a filled credential configuration form in YepCode:
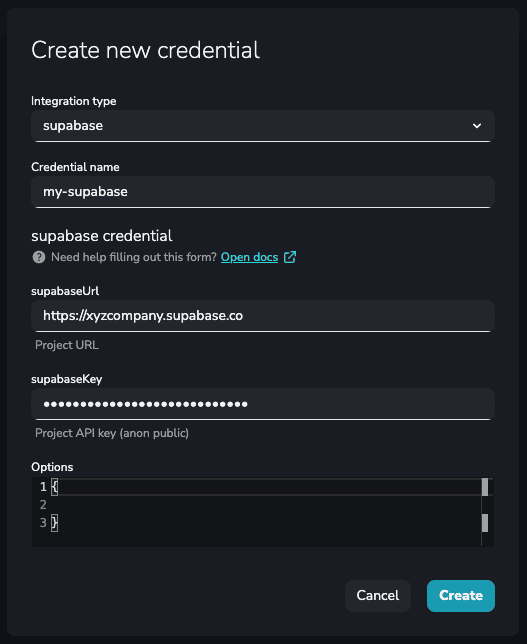
Supabase Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const supabase = yepcode.integration.supabase('credential-slug')
New integration from plain authentication data
const { createClient } = require('@supabase/supabase-js')
const supabase = createClient('your-project-url', 'your-api-token')
Fetch Data
Fetch data
const { data, error } = await supabase
.from('countries')
.select('name, country_id')
.eq('name', 'The Shire')
Insert Data
Insert data
const { error } = await supabase
.from('countries')
.insert({ id: 1, name: 'Denmark' })
Update Data
Update data
const { error } = await supabase
.from('countries')
.update({ name: 'Australia' })
.eq('id', 1)
Upsert Data
Upsert data
const { error } = await supabase
.from('countries')
.upsert({ id: 1, name: 'Albania' })
.eq('id', 1)
Delete Data
Delete data
const { error } = await supabase
.from('countries')
.delete()
.eq('id', 1)
Call a Postgres Function
Call a Postgres function
const { data, error } = await supabase.rpc('hello_world')
List all Files in a Bucket
List all files in a bucket
const { data, error } = await supabase
.storage
.from('my-files')
.list('public', {
limit: 100,
offset: 0,
sortBy: { column: 'name', order: 'asc' },
})
Upload a File
Upload a file
const textFileContent = 'the-content'
const { data, error } = await supabase
.storage
.from('my-files')
.upload('public/textFile.txt', textFileContent, {
cacheControl: '3600',
upsert: false
})
Download a File
Download a file
const { data, error } = await supabase.storage
.from("my-files")
.download("public/textFile.txt");
Comming soon
We are releasing new Python features every week