OpenAI
OpenAI is a natural language processing (NLP) library for AI. It was created by OpenAI.
TagsAIchatgptdall-efine-tuning
JavaScript
Python
NodeJS packagehttps://www.npmjs.com/package/openai
Version4.26.1
Source Codehttps://github.com/openai/openai-node
Pypi packagehttps://pypi.org/project/openai/
Version1.11.1
Source Codehttps://github.com/openai/openai-python
Network Connection needs
This integration needs network access to the server where the service is running.
See the Network access page for details about how to achieve that.
Credential configuration
To configure this credential you need your OpenAI API KEY
,
check this page to find out how to create it.
Here is an example of a filled credential configuration form in YepCode:
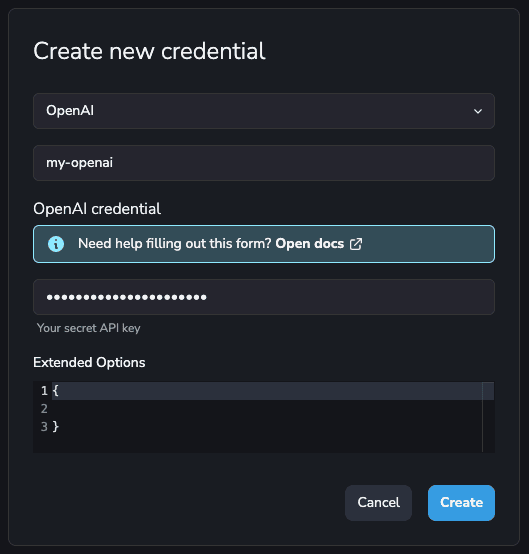
OpenAI Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const openai = yepcode.integration.openai('credential-slug');
New integration from plain authentication data
const OpenAI = require('openai');
const openai = new OpenAI({
apiKey: "OPENAI_API_KEY"
});
Completions
Create completion
const completion = await openai.completions.create({
model: 'gpt-3.5-turbo',
prompt: 'Say this is a test',
max_tokens: 7,
temperature: 0,
});
console.log(completion.choices[0].text);
Chat
Create chat completion
const completion = await openai.chat.completions.create({
model: 'gpt-3.5-turbo',
messages: [{role: "user", content: 'Hello World'}],
});
console.log(completion.choices[0].message);
Models
List models
const models = await openai.models.list();
console.log(models)
Images
Create image
const response = await openai.images.generate({
prompt: 'A cute baby sea otter',
n: 2,
size: '1024x1024',
});
console.log(response.data)
Integration
New integration from credential
openai = yepcode.integration.openai('credential-slug')
New integration from plain authentication data
from openai import OpenAI
client = OpenAI(
api_key="your-openai-api-key"
)
Completions
Create completion
completion = openai.completions.create(
model="gpt-3.5-turbo",
prompt="Say this is a test",
max_tokens=7,
temperature=0
)
print(completion.choices[0].text)
Chat
Create chat completion
chat_completion = openai.chat.completions.create(model="gpt-3.5-turbo", messages=[{"role": "user", "content": "Hello World"}])
print(chat_completion.choices[0].message.content)
Models
List models
models = openai.models.list()
print(models)
Images
Create image
response = openai.images.generate(
prompt="A cute baby sea otter",
n=2,
size="256x256"
)
print(response)