Pinecone
Pinecone is a vector database that simplifies the process of creating high-performance vector search applications
Official Websitehttps://www.pinecone.io
TagsDatabaseVector
JavaScript
Python
Documentationhttps://docs.pinecone.io/docs/node-client
Version2.0.1
Documentationhttps://docs.pinecone.io/docs/python-client
Pypi packagehttps://pypi.org/project/pinecone-client/3.0.2/
Version3.0.2
Network Connection needs
This integration needs network access to the server where the service is running.
See the Network access page for details about how to achieve that.
Credential configuration
To configure this credential, you need the environmment
, and the api key
to connect to your project.
Here is an example of a filled credential configuration form in YepCode:
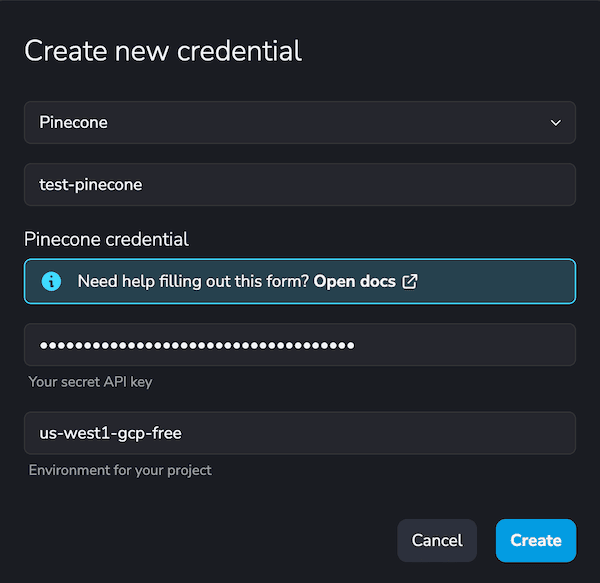
Pinecone Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const pineconeClient = yepcode.integration.pinecone('credential-slug')
New integration from plain authentication data
const { Pinecone } = require('@pinecone-database/pinecone');
const pineconeClient = new Pinecone({
apiKey: 'YOUR_API_KEY',
});
Create Index
Creates an index
await pineconeClient.createIndex({
name: "example-index",
dimension: 1024,
});
List Indexes
Lists all indexes in your project
const indexesList = await pineconeClient.listIndexes();
Construct an index Object
Construct an index objet with an existing index name
const index = pineconeClient.index('example-index');
// Now perform index operations
await index.fetch(['1']);
Describe Index
Logs information about an index in your project
const indexDescription = await pineconeClient.describeIndex({
indexName: "example-index",
});
Delete Index
Deletes an index
await pineconeClient.deleteIndex({
indexName: "example-index",
});
Upsert Vectors
Upserts vector to an index
const index = pineconeClient.index("example-index");
await index.upsert([
{
id: "vec1",
values: [0.1, 0.2, 0.3, 0.4],
metadata: {
genre: "drama",
},
},
{
id: "vec2",
values: [0.2, 0.3, 0.4, 0.5],
metadata: {
genre: "action",
},
},
]);
Query and Index
Queries an index filtering
await pineconeClient.configureIndex({
indexName: "example-index",
patchRequest: {
replicas: 2,
podType: "p2",
},
});
Update Vectors
Updates a vector
const index = pineconeClient.index("example-index");
const updateResponse = await index.update({
id: "vec1",
values: [0.1, 0.2, 0.3, 0.4],
setMetadata: { genre: "drama" },
namespace: "example-namespace",
});
Delete Vectors
Deletes a vector
const index = pineconeClient.index("example-index");
await index.deleteOne("vec1")
await index.deleteMany(["vec1", "vec2"]);
await index.deleteAll()
Create Collection
Creates a collection from an index
const createCollectionRequest = {
name: "example-collection",
source: "example-index",
};
await pineconeClient.createCollection({
createCollectionRequest,
});
List Collections
List the collections in your current project
const collectionsList = await pineconeClient.listCollections();
Describe Collection
Returns a description of one collection
const collectionDescription = await pineconeClient.describeCollection("example-collection");
Delete Collection
Deletes a collection
await pineconeClient.deleteCollection("example-collection");
Integration
New integration from credential
pineconeClient = yepcode.integration.pinecone('credential-slug')
New integration from plain authentication data
from pinecone import Pinecone
pineconeClient = Pinecone(api_key="YOUR_API_KEY")
Create Index
Creates an index
pineconeClient.create_index(name="example-index", dimension=1024)
List Indexes
List indexes in your current project
active_indexes = pineconeClient.list_indexes()
Construct an Index Object
Construct an index objet with an existing index name
index = pineconeClient.Index("example-index");
Describe Index
Describes an index
index_description = pineconeClient.describe_index("example-index")
Delete Index
Deletes an index
pineconeClient.delete_index("example-index")
Scale Replicas
Sets the number of replicas and pod type for an index
new_number_of_replicas = 4
pineconeClient.configure_index("example-index", replicas=new_number_of_replicas)
Upsert Vectors
Upserts vector to an index
index = pineconeClient.Index("example-index")
upsert_response = index.upsert(
vectors=[
(
"vec1", # Vector ID
[0.1, 0.2, 0.3, 0.4], # Dense vector values
{"genre": "drama"} # Vector metadata
),
(
"vec2",
[0.2, 0.3, 0.4, 0.5],
{"genre": "action"}
)
],
namespace="example-namespace"
)
Query an Index
Queries an index filtering
index = pineconeClient.Index("example-index")
query_response = index.query(
namespace="example-namespace",
top_k=10,
include_values=True,
include_metadata=True,
vector=[0.1, 0.2, 0.3, 0.4],
filter={
"genre": {"$in": ["comedy", "documentary", "drama"]}
}
)
Update Vector
Updates a vector
index = pineconeClient.Index("example-index")
update_response = index.update(
id="vec1",
values=[0.1, 0.2, 0.3, 0.4],
set_metadata={"genre": "drama"},
namespace="example-namespace"
)
Delete Vector
Deletes a vector
index = pineconeClient.Index("example-index")
delete_response = index.delete(ids=["vec1", "vec2"], namespace="example-namespace")
Create Collection
Creates a collection from an index
pineconeClient.create_collection(
name="example-collection",
source="example-index"
)
List Collections
List the collections from in the current project
active_collections = pineconeClient.list_collections()
Describe Collection
Returns a description of one collection
collection_description = pineconeClient.describe_collection("example-collection")
Delete Collection
Delete a collection
pineconeClient.delete_collection("example-collection")