SSH2
SSH2 gives you a secure way to access a computer over an unsecured network. This integration provides ssh2 with callback functions
Official Websitehttps://github.com/mscdex/ssh2
Tagsshell
JavaScript
Python
Documentationhttps://github.com/mscdex/ssh2
NodeJS packagehttps://www.npmjs.com/package/ssh2
Version1.11.0
Source Codehttps://github.com/mscdex/ssh2
Documentationhttps://docs.paramiko.org/en/latest/
Pypi packagehttps://pypi.org/project/paramiko/
Version3.0.0
Source Codehttps://github.com/paramiko/paramiko
Network Connection needs
This integration needs network access to the server where the service is running.
See the Network access page for details about how to achieve that.
Credential configuration
To configure this credential, you need the host
, port
and username
to connect to the SSH server.
Additionally, you'll need a password
or the private key
depending on how you want to connect.
Here is an example of a filled credential configuration form in YepCode:
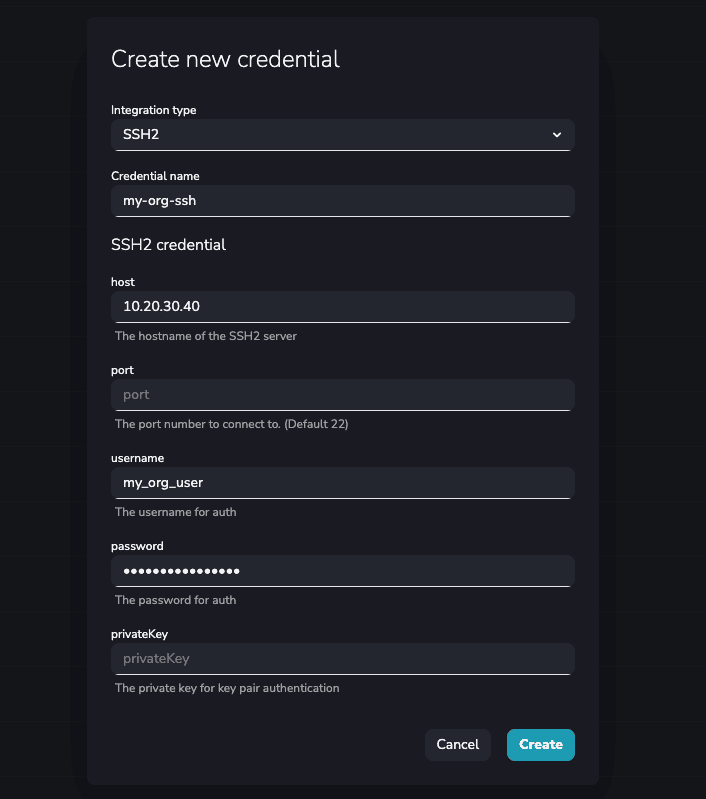
SSH2 Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const sshConnection = yepcode.integration.ssh2("credential-slug");
New integration from plain authentication data (username and password)
const { Client } = require("ssh2");
const options = {
host: "hostname",
port: portNumber,
username: "username",
password: "password",
};
const sshConnection = new Client().connect(options);
New integration from plain authentication data (private key)
const { Client } = require("ssh2");
const options = {
host: "hostname",
port: portNumber,
username: "username",
privateKey: "privateKey",
};
const sshConnection = new Client().connect(options);
Interactive Shell Session
Shell session
sshConnection.on('ready', () => {
console.log('Client :: ready');
sshConnection.shell((err, stream) => {
if (err) {
throw err;
}
stream.on('close', () => {
console.log('Stream :: close');
sshConnection.end();
}).on('data', (data) => {
console.log('OUTPUT: ' + data);
});
stream.end("your-command-here\nexit\n");
});
})
Integration
New integration from credential
ssh_connection = yepcode.integration.ssh2("credential-slug")
New integration from plain authentication data (username and password)
from paramiko.client import SSHClient
ssh_connection = SSHClient()
ssh_connection.connect(
hostname='hostname',
port='portNumber',
username='username',
password='password'
)
New integration from plain authentication data (private key)
from paramiko.client import SSHClient
ssh_connection = SSHClient()
ssh_connection.connect(
hostname='hostname',
port='portNumber',
username='username',
pkey='privateKey'
)
Interactive Shell Session
Shell session
## The command’s input and output streams are returned as Python file-like objects representing stdin, stdout, and stderr.
## Official docs: https://docs.paramiko.org/en/latest/api/client.html
ssh_stdin, ssh_stdout, ssh_stderr = ${1.ssh_connection}.exec_command('your-command-here')