How to share a Slack thread content by email
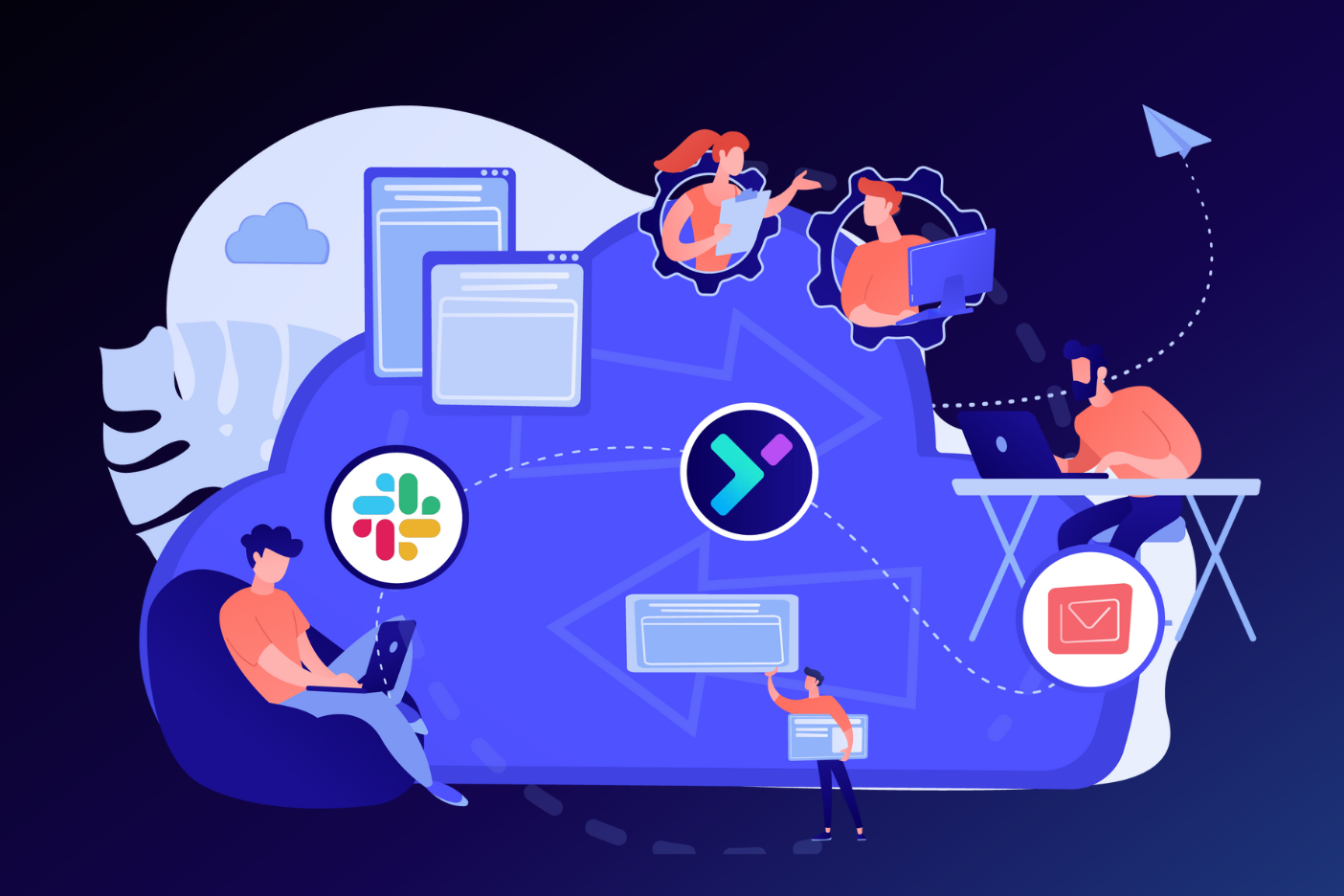
Slack is widely used in customer support teams, as its integrations with CRMs make this messaging tool the perfect way to be aware of several conversations or tickets.
Sometimes, it may be necessary to export the information generated there to share it with other teams (ie: technical support). In this post we'll show you how YepCode may help in this task, creating a process that delivers by email the content of a slack thread. To run this example we are going to need a YepCode account (it's free!) and to be a Slack admin of one workspace.
Creating the YepCode process
- Open your team workspace in YepCode
- Click on New button in the processes page
- Choose a name and a description
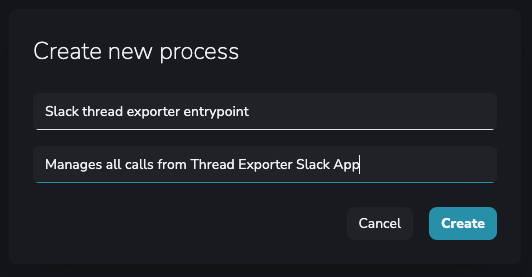
You will be redirected to the process page. Don't worry about the code of the process, we are going to deal with that later.
Now we are just going to configure a webhook on this process so Slack can communicate with it.
- Click on Add+ button in the right sidebar, next to Webhook configuration
- Don't fill basic auth options, we need this webhook to be public
- Click on Create!
Perfect! A modal will prompt you with webhook info: the important thing is the URL link. We will need it later to configure our Slack Shortcut! You always can come back to the Webhook configuration to copy the URL link.
Creating the Slack App
To create your own Slack App open your app's dashboard and click on Create New App button. You only need to choose a name and a workspace.

Excellent, we have a brand new Slack App!
We are going to use Slack Shortcuts instead of Slack Commands. Why? The reason is that you can't use commands inside a thread, instead, message shortcuts are options that are shown in any message context menu.
So... how do I create my Slack Shortcut?
- Open your app's dashboard
- Click on Interactivity & Shortcuts in the sidebar
- Enable Interactivity: It will ask you for a Request URL, paste here our webhook URL link from our YepCode process.
- Click the Create New Shortcut button under Shortcuts
- Choose On Messages option and click Next
- Fill in a name, a short description and a callback ID.
- Click that tempting green Create button, and you'll be sent back to the Interactivity & Shortcuts page
- On that page don't forget to click the Save Changes button!
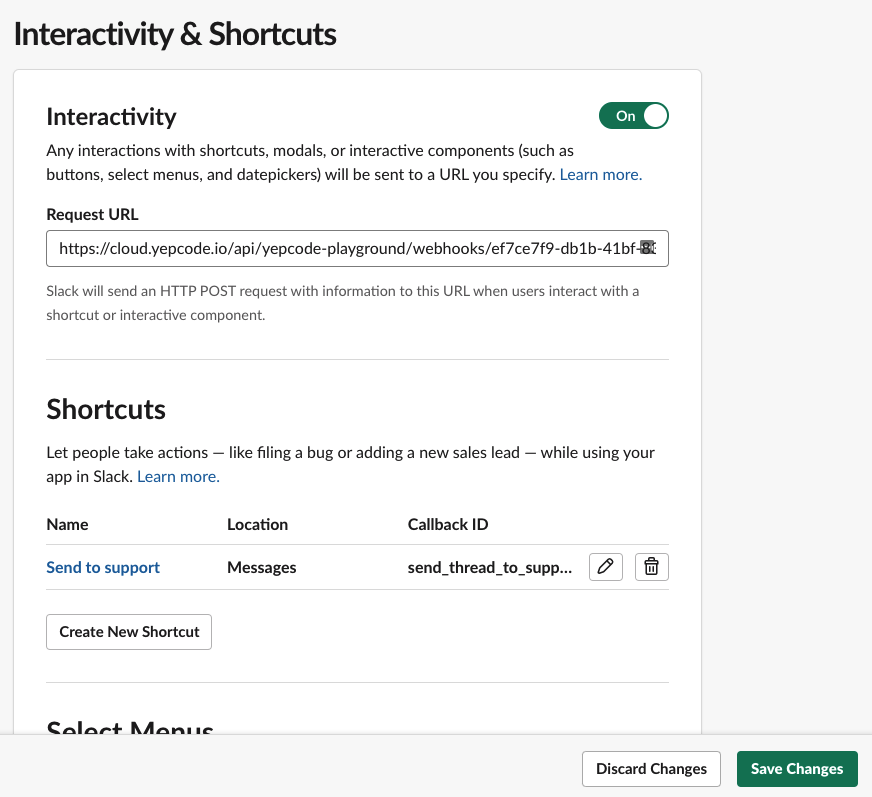
Now we need to configure an access token to communicate Slack and YepCode and add some permissions to the bot
Configure OAuth and Permissions
- Open your app's dashboard
- Click on OAuth & Permissions in the sidebar
- Click on Install on workspace button under OAuth Tokens for Your Workspace
- Click on Allow button so Slack to confirm
- You will be sent back to OAuth & Permissions where now appears a Bot User Auth Token. We are going to need it to configure a credential on YepCode.
- Scroll down in OAuth & Permissions to Bot Token Scopes section. we are going to add two new permissions: channels:history and users:read
- Reinstall the App for the changes to take effect
Implementing the Magic on YepCode
We've finished configuring our App in Slack, so let's go to YepCode to make it all work. First, we are going to create two credentials, one to store Slack tokens in a secure way and the other to store our SMTP configuration.
- Open your team workspace in YepCode
- Click on Credentials in the sidebar
- Click on New button
- Select Slack Bolt integration type
- Fill the following fields:
- Credential name: it's the unique identifier for the integration with slack i.e.: thread-exporter-slack
- Signing Secret: Grab your Slack Signing Secret, available in the app admin panel under Basic Info
- Token: This would be the Bot User Auth Token we've created on OAuth & Permissions
Click on Create and there you go! It will appear in the Credential list
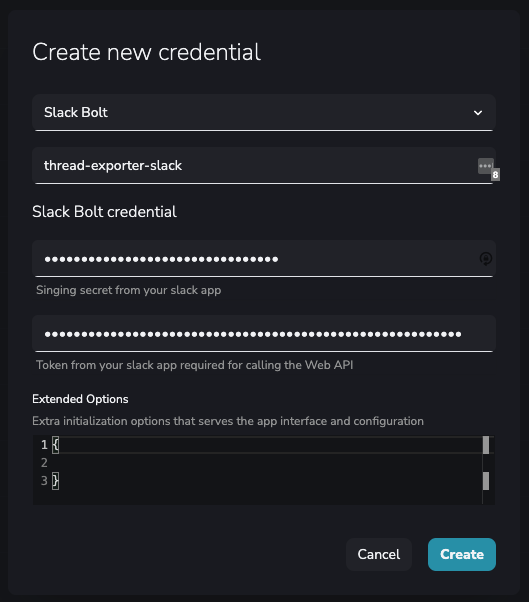
Create another credential. This time we are going to connect our SMTP so we can send mails later.
- Click again on New button on the Credentials page
- Select Nodemailer integration type
- Fill the fields with your SMTP info. You could use your Gmail account to test it.

The YepCode process
Congrats! We are close to finishing. We are going to implement the logic that will read the messages from Slack and send them to the support mail.
Navigate to the process that you created in the first step.
This is the full code, we are going to comment on some parts, but if you are feeling lucky you can execute right away! Write and save the following lines in the code editor:
To invoke this process go to your Slack workspace and click on the context menu of a message, it will appear your Shortcut, in my case is Send to support.
Important! You need to invite the App to the channel: You can do it simply by typing the following message: /invite @Thread exporter

After you click, go back to YepCode and navigate to Executions on the sidebar. It should be a new execution! Click on it and you should see something like this.
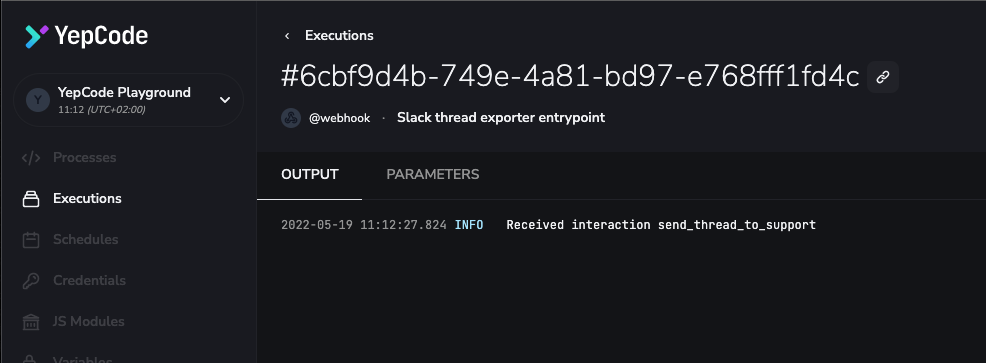
If you see something like this, congrats! You're done. If not, review the previous steps to see if something is missing.
Let's review the code!
Here we are getting the information Slack is sending to YepCode. There are a lot of fields but we only need:
- callback_id: Just to know which interaction is calling, it will match the Callback ID of the Shortcut we've configured.
- channel: the unique identifier of the Slack channel
- message_ts: the unique identifier of the Slack message that was clicked on
- message: the full info about the message that was clicked
These two lines use some YepCode magic: they initialize our integrations, the first for Nodemailer and the second for Slack Bolt. Both use their respective credentials.
We ask for the conversation messages related to our clicked message in the specified channel. If you remember we had to add a special permission channels:history in our Slack App, this is the reason.
The request returns the messages that we are going to consume.
We need some user information like username or email. It's a pity but this info is not in messages, we need to ask for it to Slack. This is the reason we added users:read permission in our Slack App. We get all userIds in messages and make a request per user saving them in the users variable.
This is the final code. We mount the email text concatenating the username and the message text. Once we have the string, we just need to use the method sendMail of Nodemailer to send it to support@example.com.
That's it! You are ready to go!
This is just a simple example of Slack Shortcuts using YepCode but we could add more logic to it. For example, it would be awesome to ask the email to send the thread, this can be done using Slack Bolt and Slack modals.
Thank you for reading :) and...
Happy coding! 😉