YepCode Forms
YepCode allows you to initiate process executions from any webpage by embedding process input parameter forms.
This feature enables each form submission to start an execution with the information filled in the form. It also allows you to manage the execution result within that webpage, including support for multistep forms!
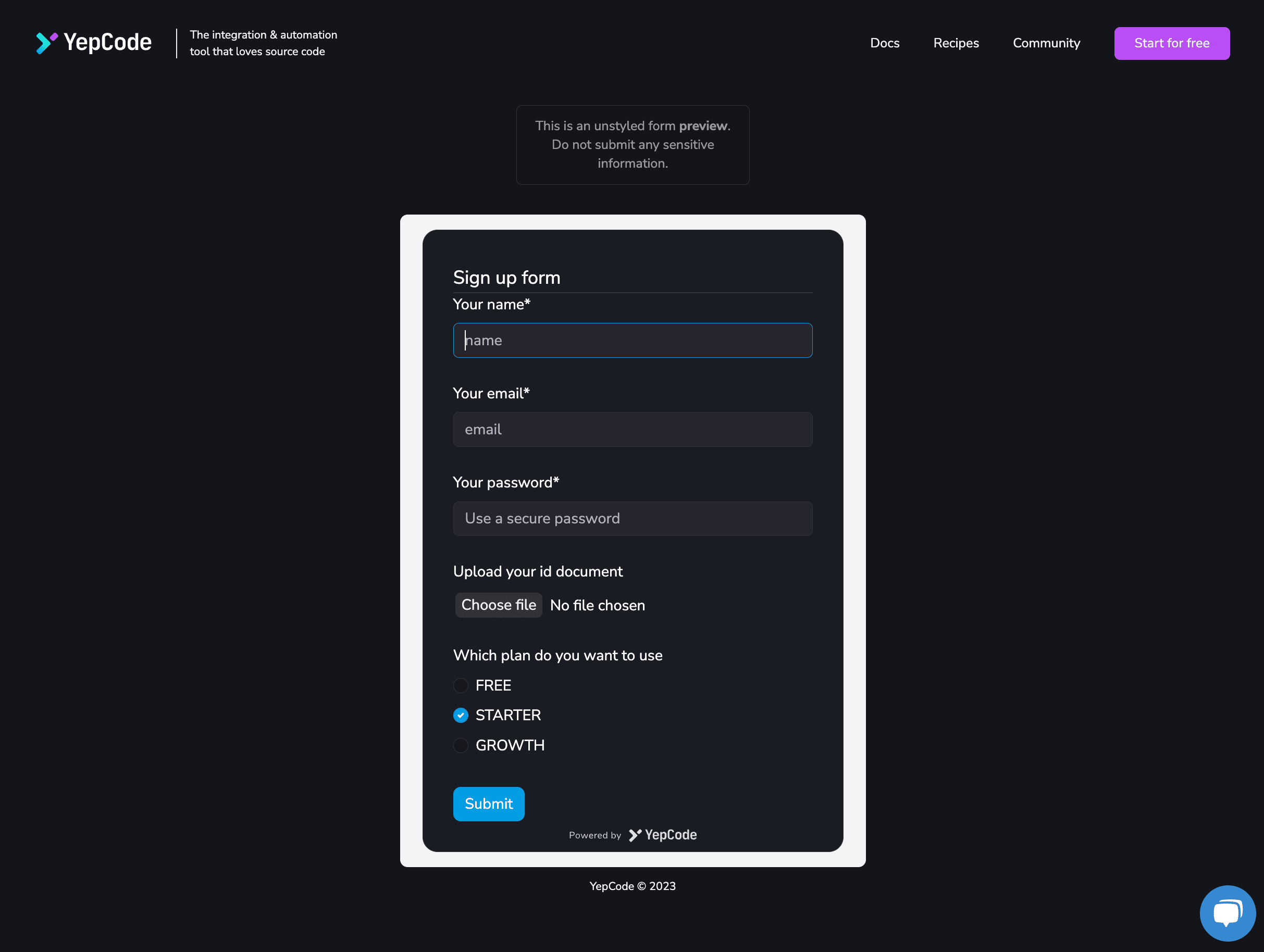
For a comprehensive demonstration of how YepCode Forms works, watch the full demo video below: