Input Parameters
When working with YepCode processes, you can configure input parameters to gather information from users before starting an on-demand or scheduled execution. Input parameters also play a role in defining webhook bodies.
The process edition includes a JSON editor which is used to define the process input parameter form.
In your process source code, you can access the provided input parameters using the following helper:
JavaScript
Python
const {
context: { parameters },
} = yepcode;
parameters = yepcode.context.parameters
With parameters defined, you can request any information needed to run your process. Forms can range from simple:
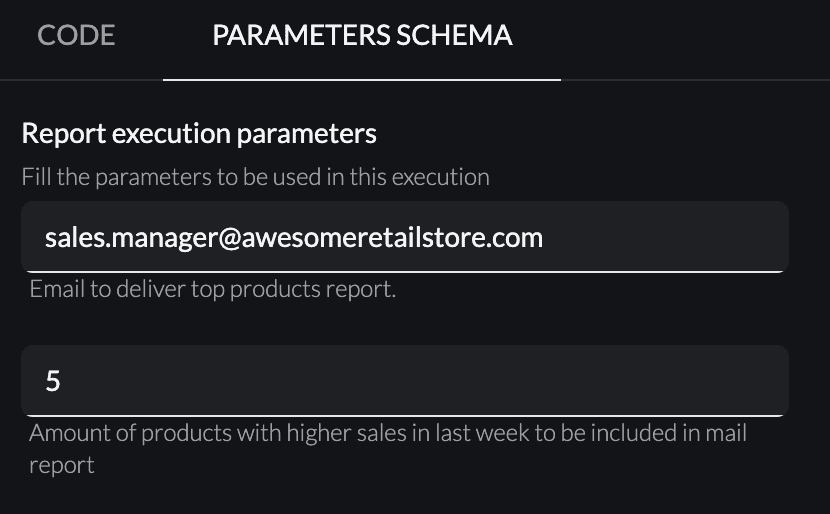
...to more complex configurations:
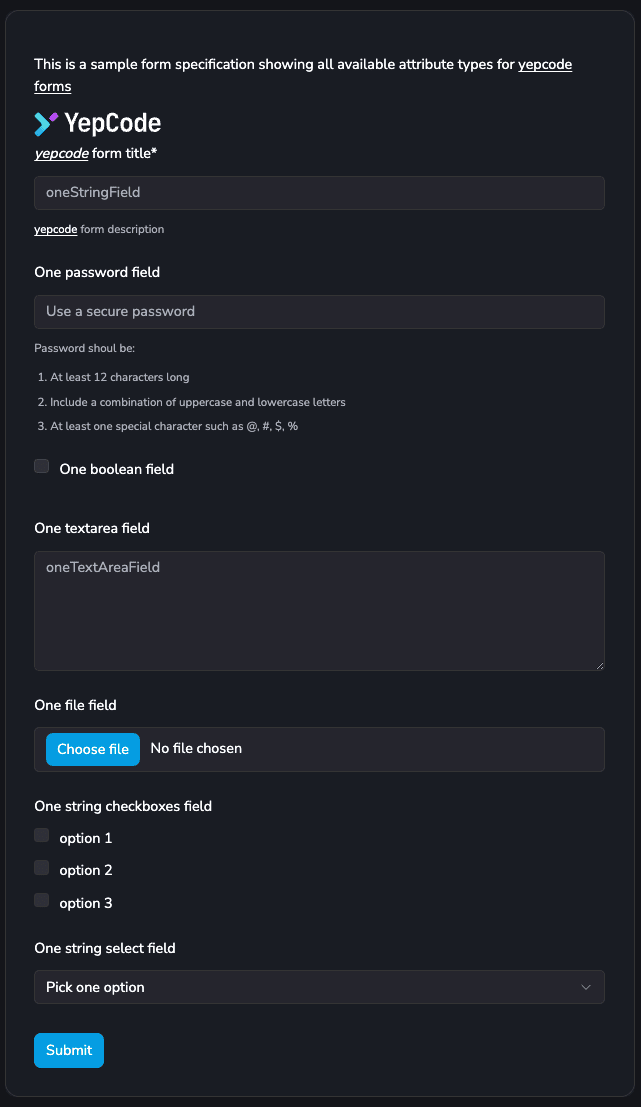
Form Builder
YepCode provides a JSON Form Builder that allows you to manage input parameters without any coding.
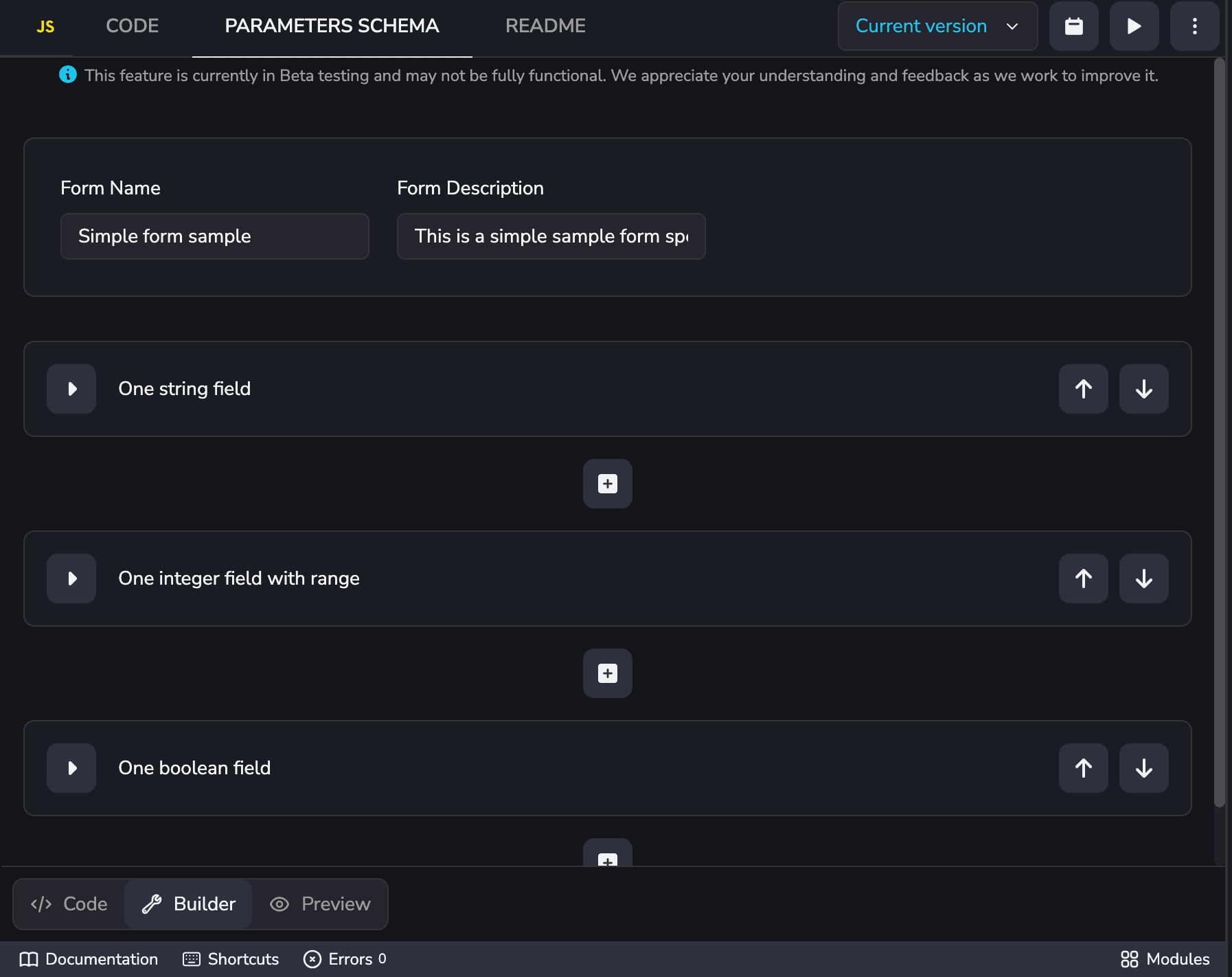
You can create new input parameters, modify existing ones, or delete unnecessary ones.
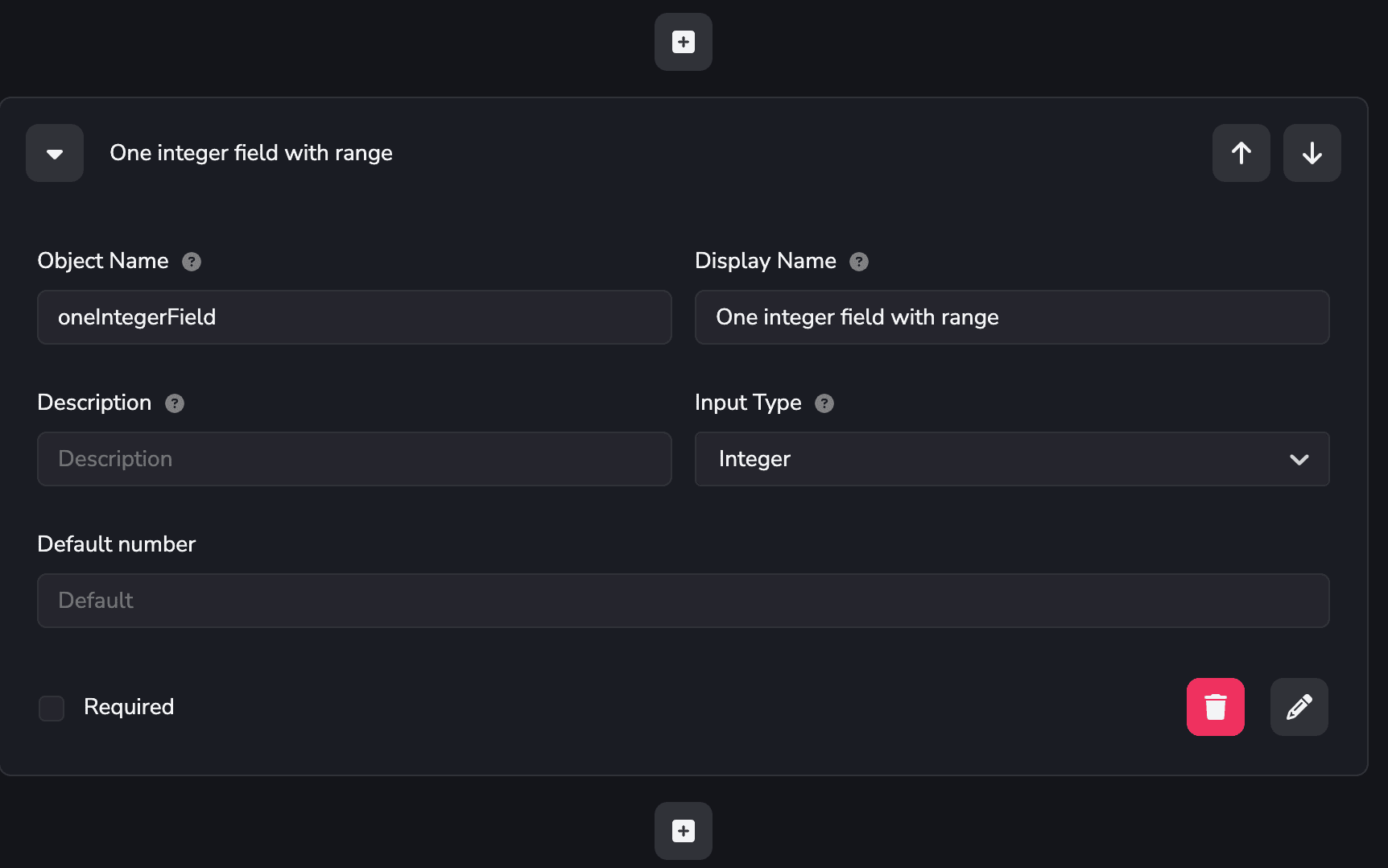
Parameters Definition
We use the JSON Schema form specification, and the library we use to render the form is react-jsonschema-form.
This allows you to use advanced configurations, such as dependencies, which enable you to change fields based on entered data.
Explore our Full form sample to discover the full range of posibilities.
Additionally, YepCode offers extra attributes to enhance your experience:
Sensitive Properties
Properties marked as sensitive are treated as passwords. They are stored encrypted in the database and won't be shown in the UI.
Here you have an example of a simple form with a sensitive property:
{
"title": "Input parameters",
"type": "object",
"properties": {
"apiKey": {
"title": "The API Key",
"type": "string",
"isSensitive": true
}
},
"required": ["apiKey"]
}
Transient properties
Transient properties are only available for the execution and won't be stored in the database. An execution which receives transient parameters cannot be rerun,
as these type of parameters won't be available. They are shown with [transient]
in the UI.
Here you have an example of a simple form with a transient property:
{
"title": "Input parameters",
"type": "object",
"properties": {
"fileContent": {
"title": "The content of the file",
"type": "string",
"isTransient": true
}
},
"required": ["fileContent"]
}
Validation
Validation is supported by this form specification and is checked upon form submission.
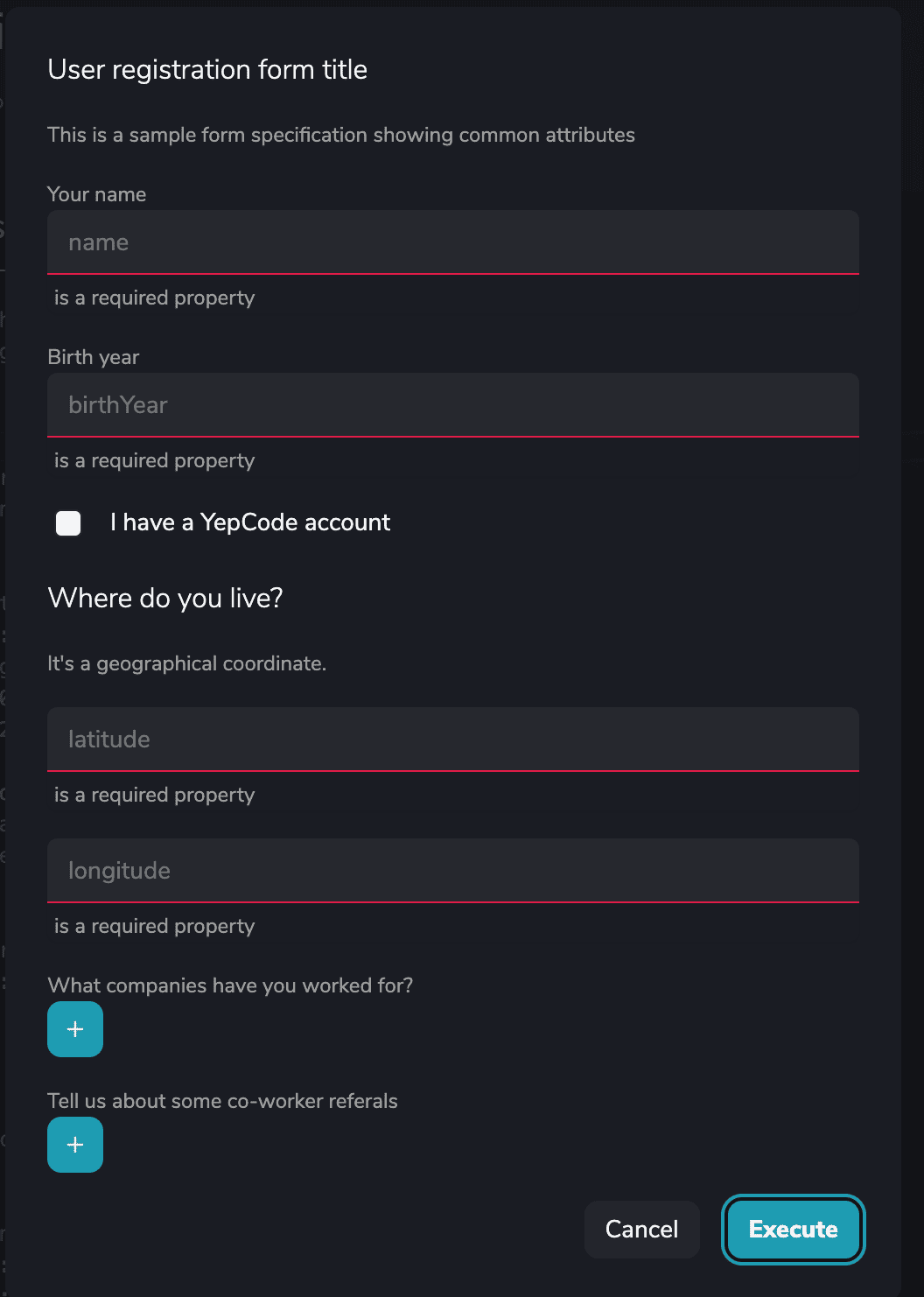
By default, validation won't be enforced if the execution starts using non-submit form methods (e.g., webhooks or API). If you want to enable this validation for all start methods, just activate the Validate parameters schema
in the settings page.
If you want to enforce validation, but for some process you want to allow additional properties, there is a flag for this behaviour.
Content Customization
To provide greater flexibility to the forms and the content they display (titles, descriptions, help messages), we have improved and extended the schema to support markdown rendering using markdown.
With this functionality, you can format text in a simple and easy way to make it more readable. Here you have one sample:
"oneStringField": {
"title": " *[yepcode](https://yepcode.io)* form title",
"description": "[yepcode](https://yepcode.io) form description",
"type": "string"
},
Full sample
Here's a complete example of a Form and its schema:
{
"title": "Full [yepcode forms](https://yepcode.io) form sample",
"description": "This is a sample form specification showing all available attribute types for [yepcode forms](https://yepcode.io)",
"type": "object",
"properties": {
"oneStringField": {
"title": " *[yepcode](https://yepcode.io)* form title",
"description": "Visit [yepcode](https://yepcode.io) form description",
"type": "string"
},
"onePasswordField": {
"title": "One password field",
"type": "string",
"description": "Password shoul be: \n 1. At least 12 characters long \n 2. Include a combination of uppercase and lowercase letters \n 3. At least one special character such as @, #, $, %",
"isSensitive": true,
"ui": {
"ui:placeholder": "Use a secure password"
}
},
"oneHiddenField": {
"title": "One hidden field",
"type": "string",
"ui": {
"ui:widget": "hidden"
}
},
"oneIntegerField": {
"title": "One integer field with range",
"description": "Values must be between 0 and 500",
"type": "integer",
"minimum": 0,
"maximum": 500
},
"oneBooleanField": {
"title": "One boolean field with [link](https://yepcode.io)",
"type": "boolean"
},
"oneEmailField": {
"title": "One email field",
"type": "string",
"format": "email"
},
"oneTextAreaField": {
"title": "One textarea field",
"type": "string",
"description": "> Block quote description",
"ui": {
"ui:widget": "textarea"
}
},
"oneColorField": {
"title": "One color field",
"type": "string",
"ui": {
"ui:widget": "color"
}
},
"oneFileField": {
"title": "One file field",
"type": "string",
"ui": {
"ui:widget": "file"
}
},
"oneObjectField": {
"title": "One object field",
"description": "This sample has two nested fields.",
"required": [
"anotherString",
"anotherInteger"
],
"type": "object",
"properties": {
"anotherString": {
"type": "string"
},
"anotherInteger": {
"type": "number",
"minimum": -180,
"maximum": 180
}
}
},
"oneStringArrayField": {
"title": "One string array field",
"type": "array",
"items": {
"type": "string"
}
},
"oneObjectsArrayField": {
"title": "One object array field",
"type": "array",
"items": {
"type": "object",
"properties": {
"oneProperty": {
"description": "One property",
"type": "string"
},
"anotherProperty": {
"description": "Another property",
"type": "string"
}
}
}
},
"oneRadioField": {
"title": "One string radio field",
"type": "string",
"ui": {
"ui:widget": "radio"
},
"oneOf": [
{
"const": "option-1",
"title": "Option 1 Label"
},
{
"const": "option-2",
"title": "Option 2 Label"
},
{
"const": "option-3",
"title": "Option 3 Label"
}
]
},
"oneCheckboxField": {
"title": "One string checkboxes field",
"type": "array",
"ui": {
"ui:widget": "checkboxes"
},
"items": {
"type": "string",
"enum": [
"option 1",
"option 2",
"option 3"
]
},
"uniqueItems": true
},
"oneSelectField": {
"title": "One string select field",
"type": "string",
"ui": {
"ui:placeholder": "Pick one option"
},
"enum": [
"option 1",
"option 2",
"option 3"
]
},
"oneJsonParameter": {
"title": "A JSON field",
"description": "Block quote description",
"type": "object",
"ui": {
"ui:field": "json"
}
},
"anotherBooleanField": {
"title": "A input that shows other inputs",
"type": "boolean"
}
},
"dependencies": {
"anotherBooleanField": {
"oneOf": [
{
"properties": {
"anotherBooleanField": {
"enum": [
true
]
},
"aDependencyValueProperty": {
"title": "This is shown when anotherBooleanField is true",
"type": "string"
}
}
},
{
"properties": {
"anotherBooleanField": {
"enum": [
false
]
},
"aDependencyValueProperty": {
"title": "This is shown when anotherBooleanField is false",
"type": "string"
}
}
}
]
}
},
"required": [
"oneStringField"
],
"ui": {
"ui:submitButtonOptions": {
"submitText": "Click me!"
}
},
"withBranding": true,
"embedFormOptions": {
"loadingOverlayContent": "Creating new user...",
"withBranding": true,
"theme": "dark",
"themeStylesheet": "",
"loadingOverlayDisabled": false,
"locale": "es"
}
}