AWS S3
With this integration you can store and access data into amazon S3 buckets.
Official Websitehttps://aws.amazon.com/s3
TagsBucketsFilesSaas
JavaScript
Python
NodeJS packagehttps://www.npmjs.com/package/@aws-sdk/client-s3
Version3.316.0
Credential configuration
To configure this credential, you need the Access Key ID
and the Secret Access Key
of the Programatic access
user you want to use. Make sure this user has the required permissions
to access S3 to work properly. If you need to create the user,
follow the instructions provided here.
In the extra options field, you can include any of the parameters found here.
Here is an example of a filled credential configuration form in YepCode:
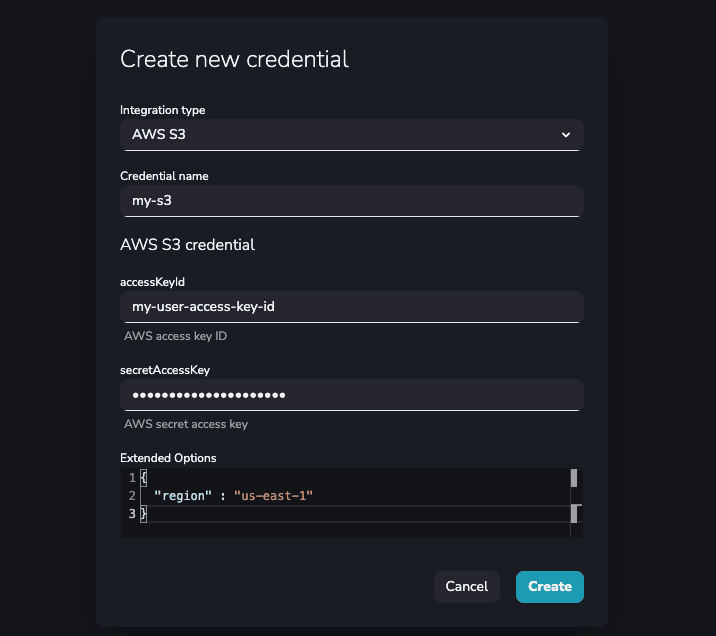
AWS S3 Snippets available in YepCode editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const awsS3Client = yepcode.integration.awsS3("credential-slug");
New integration from plain authentication data
const { S3Client } = require("@aws-sdk/client-s3");
const awsS3Client = new S3Client({
credentials: {
accessKeyId: "accessKeyId",
secretAccessKey: "secretAccessKey",
},
});
List Buckets
List buckets
const { ListBucketsCommand } = require("@aws-sdk/client-s3");
const listBucketsCommand = new ListBucketsCommand({})
const listBucketsResult = await awsS3Client.send(listBucketsCommand);
listBucketsResult.Buckets.forEach(console.log)
Create a Bucket
Create a bucket
const { CreateBucketCommand } = require("@aws-sdk/client-s3");
const createBucketCommand = new CreateBucketCommand({ Bucket: "bucket-name" });
awsS3Client.send(createBucketCommand).then(console.log).catch(console.error);
Delete a Bucket
Delete a bucket
const { DeleteBucketCommand } = require("@aws-sdk/client-s3");
const deleteBucketCommand = new DeleteBucketCommand({ Bucket: "bucket-name" });
awsS3Client.send(deleteBucketCommand).then(console.log).catch(console.error);
Get File Content
Get file content
const { GetObjectCommand } = require("@aws-sdk/client-s3");
const getObjectCommand = new GetObjectCommand({
Bucket: "bucket-name",
Key: "object-name",
});
const getObjectResult = await awsS3Client.send(getObjectCommand);
// Result.Body is a stream of object content
getObjectResult.Body.on("data", (data) => console.log(data.toString("utf8")));
Upload File
Upload file
const { PutObjectCommand } = require("@aws-sdk/client-s3");
const putObjectCommand = new PutObjectCommand({
Body: "Some string body, or stream with defined length",
Bucket: "bucket-name",
Key: "object-name",
});
awsS3Client.send(putObjectCommand).then(console.log).catch(console.error);
Upload File with Stream
Upload file with stream
const { Upload } = require("@aws-sdk/lib-storage");
const upload = new Upload({
client: awsS3Client,
params: {
Bucket: "bucket-name",
Key: "object-name",
Body: readableStream,
},
});
upload.done().then(console.log).catch(console.error);
Integration
New integration from credential
aws_s3_client = yepcode.integration.aws_s3("credential-slug")
New integration from plain authentication data
import boto3
session = boto3.Session(
aws_access_key_id="accessKeyId",
aws_secret_access_key="secretAccessKey",
region_name="region"
)
aws_s3_client = session.client("s3")
List Buckets
List buckets
response = aws_s3_client.list_buckets()
for bucket in response['Buckets']:
print(bucket)
Create a Bucket
Create a bucket
aws_s3_client.create_bucket(Bucket="bucket-name")
Delete a Bucket
Delete a bucket
aws_s3_client.delete_bucket(Bucket="bucket-name")
Get File Content
Get file content
response = aws_s3_client.get_object(
Bucket="bucket_name", Key="object_key"
)
file_stream = response['Body']
print(file_stream.read().decode('utf-8'))
Upload File
Upload file
aws_s3_client.put_object(
Bucket="bucket_name", Key="object_key", Body="object_body"
)
Upload File with Stream
Upload file with stream
import io
sample_file_content = """
Sample file content
With 2 lines
"""
content_stream = io.BytesIO()
content_stream.write(sample_file_content.encode('utf-8'))
# Reset stream position to the beginning
content_stream.seek(0)
aws_s3_client.put_object(
Bucket="bucket_name", Key="object_key", Body=content_stream
)