SFTP
SSH File Transfer Protocol (SFTP) gives you a secure file transfer capability. Wrapped around SSH2 which provides a high level convenience abstraction as well as a Promise based API.
JavaScript
Python
Comming soon
We are releasing new Python features every week
Network Connection needs
This integration needs network access to the server where the service is running.
See the Network access page for details about how to achieve that.
This integration works with SSH File Transfer Protocol (SFTP). If you need to use another FTP protocol, take a look at our FTP integration.
Credential configuration
To configure this credential, you need the host
, port
and username
to connect to the FTP server.
Then you'll need a password
or the private key
depending on how you want to connect.
Optionally, you can set any of the extra config parameters you can see here.
Here is an example of a filled credential configuration form in YepCode:
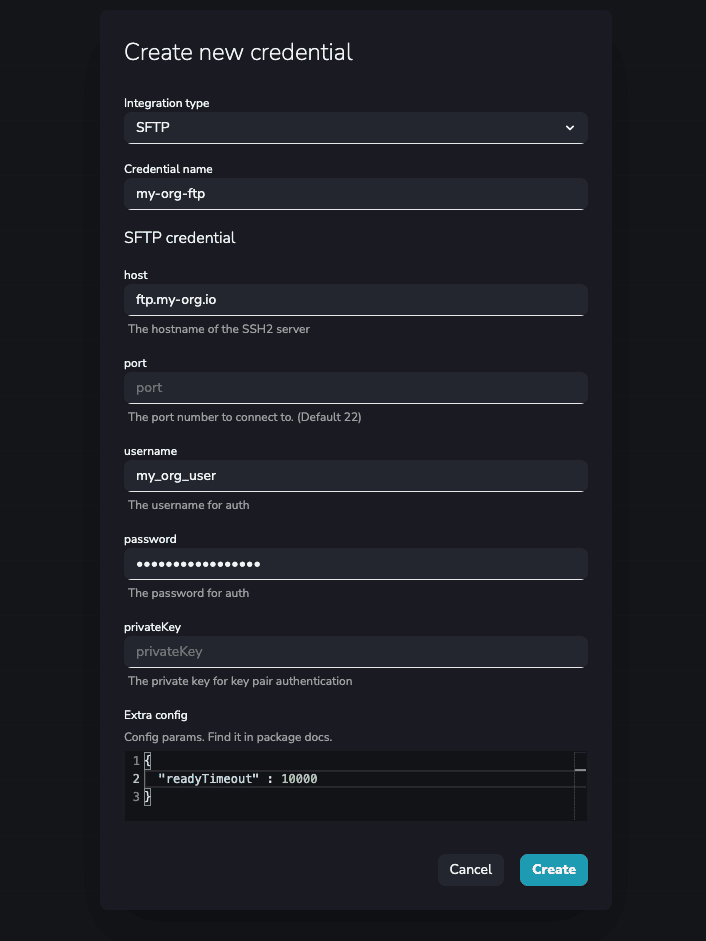
SFTP Snippets available in Editor
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
const sftpClient = await yepcode.integration.sftp('credential-slug');
const Client = require('ssh2-sftp-client');
const options = {
host: 'hostname',
port: portNumber,
username: 'username',
password: 'password',
};
const sftpClient = new Client();
await sftpClient.connect(options);
const Client = require('ssh2-sftp-client');
const options = {
host: 'hostname',
port: portNumber,
username: 'username',
privateKey: 'privateKey',
};
const sftpClient = new Client();
await sftpClient.connect(options);
Directory Listing
sftpClient.list('remoteDirPath')
.then((data) => {
console.log(data);
})
.catch(console.error)
.finally(() => sftpClient.end());
Check if Directory Exists
sftpClient.exists('remoteDirPath')
.then((exists) => {
console.log(exists);
})
.catch(console.error)
.finally(() => sftpClient.end());
Object Stats
sftpClient.stat('remoteDirPath')
.then((data) => {
console.log(data);
})
.catch(console.error)
.finally(() => sftpClient.end());
Retrieve a File
const Stream = require('stream');
const echoStream = new Stream.Writable({
write: function (chunk, encoding, next) {
console.debug('echoStream:', chunk.toString());
next();
}
});
sftpClient.get('remoteDirPath', echoStream)
.catch(console.error)
.finally(() => sftpClient.end());
Upload Data
const Stream = require('stream');
const readableStream = new Stream.Readable({
read() {
// Create your readable stream
}
});
sftpClient.put(readableStream, 'remoteDirPath')
.catch(console.error)
.finally(() => sftpClient.end());
Append Input Data
sftpClient.append(Buffer.from('Hello world'), 'remoteFilePath')
.catch(console.error)
.finally(() => sftpClient.end());
Delete a File
sftpClient.delete('remoteFilePath')
.catch(console.error)
.finally(() => sftpClient.end());
Create a New Directory
sftpClient.mkdir('remoteFilePath', true)
.catch(console.error)
.finally(() => sftpClient.end());
Rename a File or Directory
const from = '/remote/path/to/old.txt';
const to = '/remote/path/to/new.txt';
sftpClient.rename(from, to)
.catch(console.error)
.finally(() => sftpClient.end());
POSIX Rename
const from = '/remote/path/to/old.txt';
const to = '/remote/path/to/new.txt';
sftpClient.posixRename(from, to)
.catch(console.error)
.finally(() => sftpClient.end());
Current Directory
sftpClient.cwd()
.then((directory) => {
console.log(`Remote working directory is ${directory}`);
})
.catch(console.error)
.finally(() => sftpClient.end());
Comming soon
We are releasing new Python features every week