AWS Redshift
With this integration you can query and modify data from your Redshift databases.
Official Websitehttps://aws.amazon.com/redshift/
TagsdatabaseSaas
JavaScript
Python
Documentationhttps://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/clients/client-redshift-data/index.html
Version3.316.0
Documentationhttps://boto3.amazonaws.com/v1/documentation/api/latest/reference/services/redshift-data.html
Pypi packagehttps://pypi.org/project/boto3/
Version1.34.23
Source Codehttps://github.com/boto/boto3
Credential configuration
To configure this credential, you need the Access Key ID
and the Secret Access Key
of the Programatic access
user you want to use. Make sure this user has the required permissions
to access Redshift to work properly. If you need to create the user,
follow the instructions provided here.
In the extra options field, you can include any of the parameters found here.
Here is an example of a filled credential configuration form in YepCode:
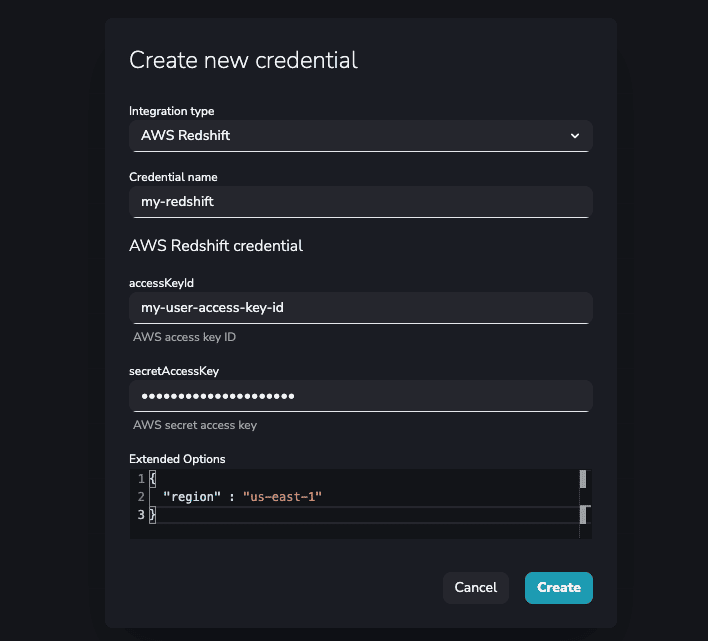
AWS Redshift Snippets available in YepCode Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const awsRedshiftClient = yepcode.integration.awsRedshift("credential-slug");
New integration from plain authentication data
const { RedshiftDataClient } = require("@aws-sdk/client-redshift-data");
const awsRedshiftClient = new RedshiftDataClient({
credentials: {
accessKeyId: "accessKeyId",
secretAccessKey: "secretAccessKey",
},
});
Execute Statement
Execute statement
const { ExecuteStatementCommand } = require("@aws-sdk/client-redshift-data");
const executeStatementCommand = new ExecuteStatementCommand({
ClusterIdentifier: "clusterIdentifier",
Database: "database",
DbUser: "dbUser",
Sql: "sql",
});
const executeStatementResult = await awsRedshiftClient.send(executeStatementCommand);
console.log(`Statement id: ${executeStatementResult.Id}`);
Execute Statement with Parameters
Execute statement with parameters
const { ExecuteStatementCommand } = require("@aws-sdk/client-redshift-data");
const executeStatementCommand = new ExecuteStatementCommand({
ClusterIdentifier: "clusterIdentifier",
Database: "database",
DbUser: "dbUser",
Sql: "your sql with params as :paramName",
Parameters: [
{
name: "paramName",
value: "value",
},
],
});
const executeStatementResult = await awsRedshiftClient.send(executeStatementCommand);
console.log(`Statement id: ${executeStatementResult.Id}`);
Get Statement Result
Get statement result
const { GetStatementResultCommand } = require("@aws-sdk/client-redshift-data");
const getStatementResultCommand = new GetStatementResultCommand({
Id: "statementId",
});
awsRedshiftClient.send(getStatementResultCommand).then(result => {
console.log(getStatementResult.Records)
})
List Statements
List statements
const { ListStatementsCommand } = require("@aws-sdk/client-redshift-data");
const listStatementsCommand = new ListStatementsCommand({});
awsRedshiftClient.send(listStatementsCommand).then(result => {
console.log(result.Statements);
});
Integration
New integration from credential
aws_redshift_client = yepcode.integration.aws_redshift("credential-slug")
New integration from plain authentication data
import boto3
session = boto3.Session(
aws_access_key_id="accessKeyId",
aws_secret_access_key="secretAccessKey",
region_name="region"
)
aws_redshift_client = session.client("redshift-data")
Execute Statement
Execute statement
response = aws_redshift_client.execute_statement(
ClusterIdentifier="cluster-name",
Database="db-name",
DbUser="db-user",
Sql="sql"
)
print(response.get("Id"))
Execute Statement with Parameters
Execute statement with parameters
sql = "select * from users where id = :id"
params = [{"name": "id", "value": "1"}]
response = aws_redshift_client.execute_statement(
ClusterIdentifier="cluster-name",
Database="db-name",
DbUser="db-user",
Sql=sql,
Parameters=params
)
print(response.get("Id"))
Describe Statement
Describe statement
response = aws_redshift_client.describe_statement(Id="statement-id")
print(response.get("Status"))
Get Statement Result
Get statement result
response = aws_redshift_client.get_statement_result(Id="statement-id")
for record in response.get("Records", []):
print(record)
List Statements
List statements
result = aws_redshift_client.list_statements()
for statement in result.get("Statements", []):
print(statement)