Notion
Notion is a project management and note-taking software
Official Websitehttps://www.notion.so
Tagsproductivitynotesdocstasks
JavaScript
Python
Documentationhttps://developers.notion.com/
NodeJS packagehttps://www.npmjs.com/package/@notionhq/client
Version2.2.14
Source Codehttps://github.com/makenotion/notion-sdk-js
Comming soon
We are releasing new Python features every week
Credential configuration
To configure this credential, you only need to create an integration
to use the Notion API and set the Internal integration token
as auth
in this form.
Optionally, you can set any of the extra config parameters you can see here.
Here is an example of a filled credential configuration form in YepCode:
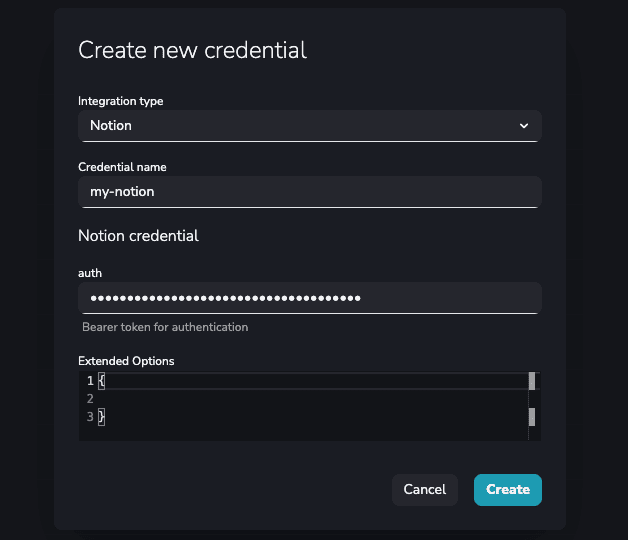
Notion Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const notion = yepcode.integration.notion('yepcode-notion')
New integration from plain authentication data
const { Client } = require('@notionhq/client')
const notion = new Client({
auth: 'NOTION_TOKEN'
})
Users
Get users
await notion.users.list({}).then(({ results }) => {
console.log(results)
})
Databases
Get a list of pages
await notion.databases
.query({
database_id: '9bc30ad4-9373-46a5-84ab-0a7845ee52e6',
filter: {
or: [
{
property: 'In stock',
checkbox: {
equals: false
}
}
]
},
sorts: [
{
property: 'Last ordered',
direction: 'ascending'
}
]
})
.then(console.log)
Create a database
const database = await notion.databases.create({
parent: {
type: 'page_id',
page_id: '98ad959b-2b6a-4774-80ee-00246fb0ea9b'
},
icon: {
type: 'emoji',
emoji: '🎉'
},
cover: {
type: 'external',
external: {
url: 'https://website.domain/images/image.png'
}
},
title: [
{
type: 'text',
text: {
content: 'Grocery List',
link: null
}
}
],
properties: {
Name: {
title: {}
},
Description: {
rich_text: {}
},
'In stock': {
checkbox: {}
},
'Food group': {
select: {
options: [
{
name: '🥦Vegetable',
color: 'green'
},
{
name: '🍎Fruit',
color: 'red'
},
{
name: '💪Protein',
color: 'yellow'
}
]
}
},
Price: {
number: {
format: 'dollar'
}
},
'Last ordered': {
date: {}
},
'Store availability': {
type: 'multi_select',
multi_select: {
options: [
{
name: 'Duc Loi Market',
color: 'blue'
},
{
name: 'Rainbow Grocery',
color: 'gray'
},
{
name: 'Nijiya Market',
color: 'purple'
},
{
name: "Gus'''s Community Market",
color: 'yellow'
}
]
}
},
'+1': {
people: {}
},
Photo: {
files: {}
}
}
})
Pages
Create a page
const response = await notion.pages.create({
parent: {
database_id: 'd9824bdc84454327be8b5b47500af6ce'
},
icon: {
type: 'emoji',
emoji: '🥬'
},
cover: {
type: 'external',
external: {
url: 'https://upload.wikimedia.org/wikipedia/commons/6/62/Tuscankale.jpg'
}
},
properties: {
Name: {
title: [
{
text: {
content: 'Tuscan Kale'
}
}
]
},
Description: {
rich_text: [
{
text: {
content: 'A dark green leafy vegetable'
}
}
]
},
'Food group': {
select: {
name: '🥦 Vegetable'
}
},
Price: {
number: 2.5
}
},
children: [
{
object: 'block',
type: 'heading_2',
heading_2: {
rich_text: [
{
type: 'text',
text: {
content: 'Lacinato kale'
}
}
]
}
},
{
object: 'block',
type: 'paragraph',
paragraph: {
rich_text: [
{
type: 'text',
text: {
content:
'Lacinato kale is a variety of kale with a long tradition in Italian cuisine, especially that of Tuscany. It is also known as Tuscan kale, Italian kale, dinosaur kale, kale, flat back kale, palm tree kale, or black Tuscan palm.',
link: {
url: 'https://en.wikipedia.org/wiki/Lacinato_kale'
}
}
}
]
}
}
]
})
console.log(response)
Blocks
Delete a block (including page blocks)
const response = await notion.blocks.delete({
block_id: '9bc30ad4-9373-46a5-84ab-0a7845ee52e6'
})
console.log(response)
Comming soon
We are releasing new Python features every week