Cassandra
With this integration you can connect to an Apache Cassandra database.
Official Websitehttps://cassandra.apache.org/_/index.html
TagsDatabase
JavaScript
Python
Documentationhttps://github.com/datastax/nodejs-driver/tree/master#datastax-nodejs-driver-for-apache-cassandra
NodeJS packagehttps://www.npmjs.com/package/cassandra-driver
Version4.7.2
Pypi packagehttps://pypi.org/project/cassandra-driver/
Version3.29.0
Source Codehttp://github.com/datastax/python-driver
Credential configuration
Fill in the appropiate values for your Cassandra connection. Specify the required fields: contactPoints
, localDataCenter
and keyspace
.
If necessary, set your username
and password
for access.
Here is an example of a filled credential configuration form in YepCode:
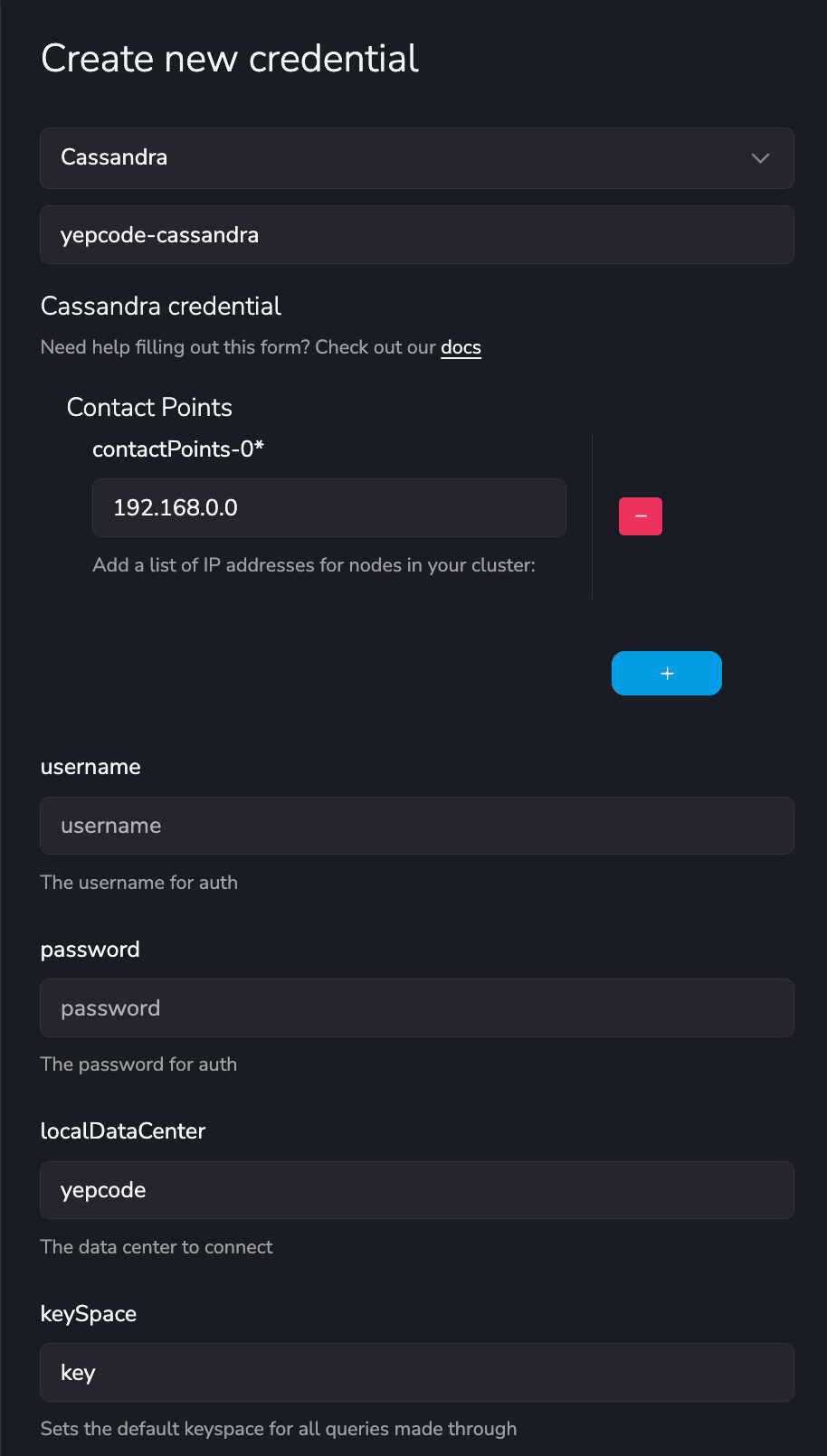
Cassandra Snippets available in YepCode editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const cassandraClient = yepcode.integration.cassandra("credential-slug");
New integration from plain authentication data
const cassandra = require('cassandra-driver');
const cassandraClient = new cassandra.Client({
contactPoints: ['h1', 'h2'],
localDataCenter: 'datacenter1',
keyspace: 'ks1'
});
New integration with authentication data
const cassandra = require('cassandra-driver');
const authProvider = new cassandra.auth.PlainTextAuthProvider(
"username",
"password"
);
const cassandraClient = new cassandra.Client({
contactPoints,
authProvider,
localDataCenter,
keyspace
});
Executes a Query
Executes a query
const query = 'SELECT name, email FROM users WHERE key = ?';
cassandraClient.execute(query, [ 'someone' ])
.then(result => console.log('User with email %s', result.rows[0].email));
Prepared Statements
Prepared statements
// Use query markers (?) and parameters
const query = 'UPDATE users SET birth = ? WHERE key=?';
const params = [ new Date(1942, 10, 1), 'jimi-hendrix' ];
// Set the prepare flag in the query options
await cassandraClient.execute(query, params, { prepare: true });
Execute Concurrent Executions
Execute concurrent executions
await cassandraClient.execute(`CREATE KEYSPACE IF NOT EXISTS examples
WITH replication = {'class': 'SimpleStrategy', 'replication_factor': '1' }`);
await cassandraClient.execute(`USE examples`);
await cassandraClient.execute(`CREATE TABLE IF NOT EXISTS tbl_sample_kv (id uuid, value text, PRIMARY KEY (id))`);
// The maximum amount of async executions that are going to be launched in parallel
// at any given time
const concurrencyLevel = 32;
// Use an Array with 10000 different values
const values = Array.from(new Array(10000).keys()).map(x => [ Uuid.random(), x.toString() ]);
const query = 'INSERT INTO tbl_sample_kv (id, value) VALUES (?, ?)';
await executeConcurrent(client, query, values);
Paging
Paging
const queries = [
{
query: 'UPDATE user_profiles SET email=? WHERE key=?',
params: [ emailAddress, 'hendrix' ]
}, {
query: 'INSERT INTO user_track (key, text, date) VALUES (?, ?, ?)',
params: [ 'hendrix', 'Changed email', new Date() ]
}
];
await cassandraClient.batch(queries, { prepare: true });
Mapper
Mapper
const cassandra = require('cassandra-driver');
const Mapper = cassandra.mapping.Mapper;
const mapper = new Mapper(cassandraClient, {
models: { 'Video': { tables: ['videos'] } }
});
// Contains all the logic to retrieve and save objects from and to the database
const videoMapper = mapper.forModel('Video');
Integration
New integration from credential
cassandra_client = yepcode.integration.cassandra("credential-slug")
New integration from plain authentication data
from cassandra.cluster import Cluster
cassandra_client = Cluster(['192.168.0.1', '192.168.0.2'])
#The connect() method takes an optional keyspace argument which sets the default keyspace for all queries made through that Session
session = cluster.connect('mykeyspace')
New integration with authentication data
from cassandra.cluster import Cluster, PlainTextAuthProvider
auth_provider = PlainTextAuthProvider(username="username", password="password")
cassandra_client = Cluster(['192.168.0.1', '192.168.0.2'], auth_provider=auth_provider)
#The connect() method takes an optional keyspace argument which sets the default keyspace for all queries made through that Session
session = cluster.connect('mykeyspace')
Executes a Query
Executes a query
rows = cassandra_client.execute('SELECT name, age, email FROM users')
for user_row in rows:
print(user_row.name, user_row.age, user_row.email)
Prepared Statements
Prepared Statements
user_lookup_stmt = cassandra_client.prepare("SELECT * FROM users WHERE user_id=?")
users = []
for user_id in user_ids_to_query:
user = session.execute(user_lookup_stmt, [user_id])
users.append(user)
Execute Concurrent Executions
Delete container
cassandra_client.execute(("CREATE KEYSPACE IF NOT EXISTS examples "
"WITH replication = {'class': 'SimpleStrategy', 'replication_factor': '1' }"))
cassandra_client.execute("USE examples")
cassandra_client.execute("CREATE TABLE IF NOT EXISTS tbl_sample_kv (id uuid, value text, PRIMARY KEY (id))")
cassandra_client = session.prepare("INSERT INTO tbl_sample_kv (id, value) VALUES (?, ?)")
for i in range(TOTAL_QUERIES):
cassandra_client.execute_async(prepared_insert, (uuid.uuid4(), str(i)))
Paging
Paging
from cassandra.query import SimpleStatement
query = "SELECT * FROM users"
statement = SimpleStatement(query, fetch_size=10)
results = cassandra_client.execute(statement)
# save the paging_state somewhere and return current results
web_session['paging_state'] = results.paging_state
# resume the pagination sometime later...
statement = SimpleStatement(query, fetch_size=10)
ps = web_session['paging_state']
results = session.execute(statement, paging_state=ps)