Oracle
This package supports basic and advanced features of Oracle Database and Oracle Client
Official Websitehttps://www.oracle.com/
TagsDatabaseSQL
JavaScript
Python
Documentationhttps://oracle.github.io/node-oracledb/doc/api.html
NodeJS packagehttps://www.npmjs.com/package/oracledb
Version5.4.0
Source Codehttps://github.com/oracle/node-oracledb
Documentationhttps://python-oracledb.readthedocs.io/en/latest/
Pypi packagehttps://pypi.org/project/oracledb/
Version2.0.1
Source Codehttps://github.com/oracle/python-oracledb
Network Connection needs
This integration needs network access to the server where the service is running.
See the Network access page for details about how to achieve that.
Credential configuration
To configure this credential, you need the connectionString
, username
and password
to connect to oracle DB.
Check out examples of connection strings here.
Optionally, you can configure additional parameters a outlined here.
Here is an example of a filled credential configuration form in YepCode:
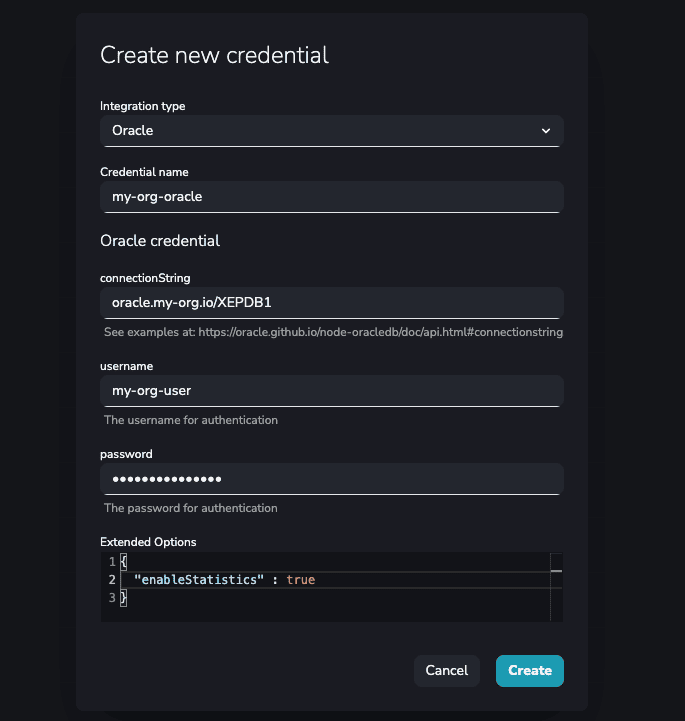
Oracle Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const oraclePool = await yepcode.integration.oracle('credential-slug')
New integration from plain authentication data
const oracledb = require("oracledb");
const oraclePool = await oracledb.createPool({
username: "username",
password: "password",
connectionString: "connectionString",
...extendedOptions,
});
Close Pool
Close pool
await oraclePool.close().catch(console.error);
Get Connection from Pool
Get connection from pool
const connection = await oraclePool.getConnection();
Close Connection
Close connection
await connection.close().catch(console.error);
SELECT Text Only
SELECT text only (async/await)
try {
const result = await connection.execute(
`SELECT id, name, email FROM Persons`
);
result.rows.forEach(console.log);
} catch (error) {
console.error(error);
}
SELECT text only (promise)
connection
.execute(`SELECT id, name, email FROM Persons`)
.then((result) => {
result.rows.forEach(console.log);
})
.catch(console.error);
SELECT Parametrized with Array
SELECT parametrized with array (async/await)
try {
const result = await connection.execute(
`SELECT * FROM Persons where firstName = :0 and lastName = :1`,
["firstName", "lastName"]
);
result.rows.forEach(console.log);
} catch (error) {
console.error(error);
}
SELECT parametrized with array (promise)
connection
.execute(`SELECT * FROM Persons where firstName = :0 and lastName = :1`, [
"firstName",
"lastName",
])
.then((result) => {
result.rows.forEach(console.log);
})
.catch(console.error);
SELECT Parametrized with Object
SELECT parametrized with object (async/await)
try {
const result = await connection.execute(
`SELECT * FROM Persons where firstName = :firstName and lastName = :lastName`,
{
firstName: "firstName",
lastName: "lastName",
}
);
result.rows.forEach(console.log);
} catch (error) {
console.error(error);
}
SELECT parametrized with object (promise)
connection
.execute(
`SELECT * FROM Persons where firstName = :firstName and lastName = :lastName`,
{
firstName: "firstName",
lastName: "lastName",
}
)
.then((result) => {
result.rows.forEach(console.log);
})
.catch(console.error);
INSERT Text Only
INSERT text only (async/await)
try {
await connection.execute(
`INSERT INTO Persons values (id, 'theName', 'theEmail')`
);
await connection.commit();
} catch (error) {
console.error(error);
}
INSERT text only (promise)
connection
.execute(`INSERT INTO Persons values (id, 'theName', 'theEmail')`)
.then((result) => {
return connection.commit();
})
.catch(console.error);
INSERT Parametrized with Array
INSERT parametrized with array (async/await)
try {
await connection.execute(`INSERT INTO Persons values (:0, :1, :2)`, [
"1",
"name",
"email",
]);
await connection.commit();
} catch (error) {
console.error(error);
}
INSERT parametrized with array (promise)
connection
.execute(`INSERT INTO Persons values (:0, :1, :2)`, ["1", "name", "email"])
.then((result) => {
return connection.commit();
})
.catch(console.error);
INSERT Parametrized with Object
INSERT parametrized with object (async/await)
try {
await connection.execute(`INSERT INTO Persons values (:id, :name, :email)`, {
id: 1,
name: "name",
email: "email",
});
await connection.commit();
} catch (error) {
console.error(error);
}
INSERT parametrized with object (promise)
connection
.execute(`INSERT INTO Persons values (:id, :name, :email)`, {
id: 1,
name: "name",
email: "email",
})
.then((result) => {
return connection.commit();
})
.catch(console.error);
Integration
New integration from credential
oracle_pool = yepcode.integration.oracle('credential-slug')
New integration from plain authentication data
import oracledb
oracle_pool = oracledb.create_pool(
user='username',
password='password',
dsn='connectionString'
)
Close Pool
Close pool
oracle_pool.close()
Get connection from pool
Get connection from pool
connection = oracle_pool.acquire()
Close Connection
Close connection
connection.close()
SELECT Text Only
SELECT text only
cursor = connection.cursor()
try:
cursor.execute('SELECT id, name, email FROM Persons')
row = cursor.fetchone()
while row:
print(row)
row = cursor.fetchone()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()
SELECT Parametrized with Array
SELECT parametrized with array
cursor = connection.cursor()
try:
cursor.execute(
'SELECT * FROM Persons where firstName = :0 and lastName = :1',
['firstName', 'lastName']
)
row = cursor.fetchone()
while row:
print(row)
row = cursor.fetchone()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()
SELECT Parametrized with Dict
SELECT parametrized with object (async/await)
cursor = connection.cursor()
try:
cursor.execute(
'SELECT * FROM Persons where firstName = :firstName and lastName = :lastName',
{
firstName: 'firstName',
lastName: 'lastName'
}
)
row = cursor.fetchone()
while row:
print(row)
row = cursor.fetchone()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()
INSERT Text Only
INSERT text only
cursor = connection.cursor()
try:
cursor.execute('INSERT INTO Persons values (id, 'theName', 'theEmail')')
connection.commit()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()
INSERT Parametrized with Array
INSERT parametrized with array
cursor = connection.cursor()
try:
cursor.execute('INSERT INTO Persons values (:0, :1, :2)', [
'1',
'name',
'email'
])
connection.commit()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()
INSERT Parametrized with Dict
INSERT parametrized with dict
cursor = connection.cursor()
try:
cursor.execute('INSERT INTO Persons values (:id, :name, :email)', {
id: 1,
name: 'name',
email: 'email'
})
connection.commit()
except (oracledb.IntegrityError as error):
print(error)
cursor.close()