Slack
JavaScript
Python
Documentationhttps://slack.dev/bolt-js/tutorial/getting-started
NodeJS packagehttps://www.npmjs.com/package/@slack/bolt
Version3.12.2
Source Codehttps://github.com/slackapi/bolt-js
Pypi packagehttps://pypi.org/project/slack-bolt/
Version1.18.1
Source Codehttps://github.com/slackapi/bolt-python
Credential configuration
To configure this credential, you need the signingSecret
and the Bolt token of your Slack app.
You can see how to create an app and find the needed secrets here.
Optionally, you can set any of the extra config parameters you can see here.
Here is an example of a filled credential configuration form in YepCode:
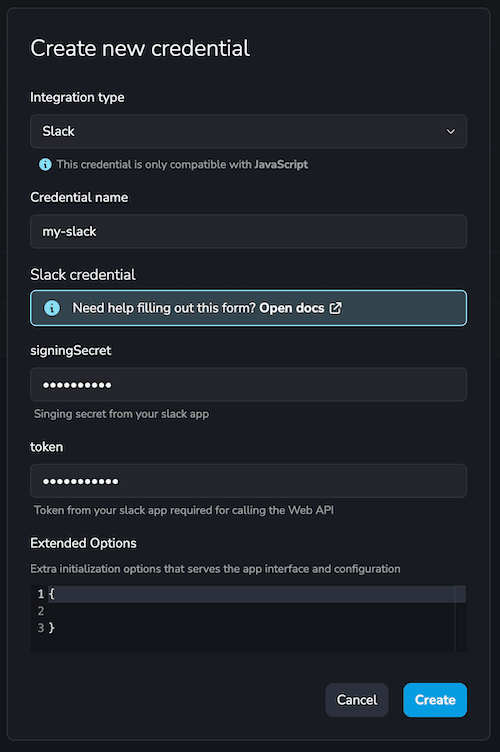
Slack Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
Excluding the Web API, the rest of the Slack Bolt features have no place in YepCode due its nature.
JavaScript
Python
Integration
New integration from credential
const app = await yepcode.integration.slack("credential-slug")
New integration from plain authentication data
const { App } = require("@slack/bolt")
const app = new App({
token: "my-token"
signingSecret: "my-signing-secret"
})
Using the Web API
Lists channels
const listChannels = async () => {
try {
const { channels } = await app.client.conversations.list();
console.log(result);
} catch (error) {
console.error(error);
}
};
listChannels();
Retrieve messages
const retrieveChannelMessages = async () => {
try {
const result = await app.client.conversations.history({
channel: "channel",
});
const { messages } = result.messages;
console.log(messages);
} catch (error) {
console.error(error);
}
};
retrieveChannelMessages();
Post a message
const postMessage = async () => {
try {
const result = await app.client.chat.postMessage({
channel: "channel",
text: "He who controls the Spice, controls the universe!",
});
console.log(result);
} catch (error) {
console.error(error);
}
};
postMessage();
Upload a file
const { Readable } = require("stream");
// You can use any read stream to upload a file
const readStream = new Readable({
read() {
this.push("Sample stream data");
this.push(null);
},
});
const uploadAFile = async () => {
try {
const result = await app.client.files.uploadV2({
channels: "channel",
initial_comment: "Here's my file :smile:",
filename: "filename.txt",
file: readStream,
});
console.log(result);
} catch (error) {
console.error(error);
}
};
uploadAFile();
Integration
New integration from credential
app = yepcode.integration.slack("credential-slug")
New integration from plain authentication data
from slack_bolt import App
app = App(token="your-token", signing_secret="your-signing-secret")
Using the Web API
Lists channels
channels = app.client.conversations_list()
print(channels)
Retrieve messages
result = app.client.conversations_history(
channel="channel_id"
)
messages = result["messages"]
print(messages)
Post a message
app.client.chat_postMessage(
channel="channel_id",
text="Test bot message!"
)
Upload a file
import io
file_content = """
Some multiline file content
Other line
"""
app.client.files_upload_v2(
channel="channel_id",
initial_comment="Here's the file :smile:",
file=io.BytesIO(file_content.encode("utf-8")),
filename="filename.txt"
)