Snowflake
Snowflake is the only data platform built for the cloud for all your data and all your users.
Official Websitehttps://www.snowflake.com/
TagsDatabaseSaas
JavaScript
Python
NodeJS packagehttps://www.npmjs.com/package/snowflake-sdk
Version1.6.23
Version3.6.0
Credential configuration
To configure this credential, you need the account
, username
, password
, database
and schema
to connect to mysql.
Optionally, you can set any of the extra config parameters you can see here.
Here is an example of a filled credential configuration form in YepCode:
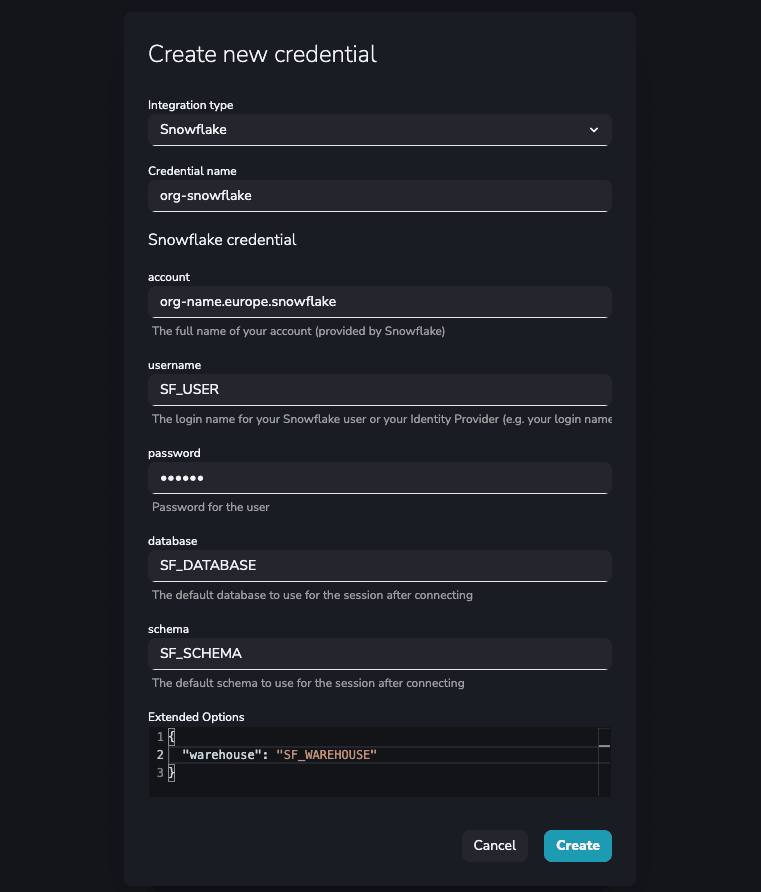
Snowflake Snippets available in Editor
note
The title is the triggering text for YepCode to autocomplete the script.
JavaScript
Python
Integration
New integration from credential
const snowflakeClient = yepcode.integration.snowflake('credential-slug')
New integration from plain authentication data
const snowflake = require('snowflake-sdk')
const snowflakeClient = snowflake.createConnection({
account: account,
username: username,
password: password
});
Connect
Establish connection
snowflakeClient.connect(
function(err, conn) {
if (err) {
console.error('Unable to connect: ' + err.message);
} else {
console.log('Successfully connected to Snowflake.');
}
}
);
Execute Statement
Execute statement
const statement = snowflakeClient.execute({
sqlText: "create database testdb",
complete: function (err, stmt, rows) {
if (err) {
console.error(
"Failed to execute statement due to the following error: " + err.message
);
} else {
console.log("Successfully executed statement: " + statement.getSqlText());
}
},
});
Execute Statement with Parameters
Execute statement with binding statement parameters
const statement = snowflakeClient.execute({
sqlText:
"select c1 from (select :1 as c1 union all select :2 as c1) where c1 = :1;",
binds: [1, 2],
complete: function (err, stmt, rows) {
if (err) {
console.error(
"Failed to execute statement due to the following error: " + err.message
);
} else {
console.log("Successfully executed statement: " + statement.getSqlText());
}
},
});
Inline Results
Select returning results inline
snowflakeClient.execute({
sqlText: "select PRODUCT_ID from PRODUCTS",
complete: function (err, stmt, rows) {
if (err) {
console.error(
"Failed to execute statement due to the following error: " + err.message
);
} else {
console.log("Number of rows produced: " + rows.length);
}
rows.forEach((row) => {
console.log("Product id ", row.PRODUCT_ID)
})
},
});
Streaming Results
Select streaming results
const statement = snowflakeClient.execute({
sqlText: "select PRODUCT_ID from PRODUCTS",
});
const stream = statement.streamRows();
stream.on('error', function(err) {
console.error('Unable to consume all rows');
});
stream.on('data', function(row) {
console.log("Product id ", row.PRODUCT_ID)
});
stream.on('end', function() {
console.log('All rows consumed');
});
Destroy Connection
Terminating a connection
snowflakeClient.destroy(function (err, conn) {
if (err) {
console.error("Unable to disconnect: " + err.message);
} else {
console.log("Disconnected connection with id: " + snowflakeClient.getId());
}
});
Integration
New integration from credential
snowflake_connection = yepcode.integration.snowflake('credential-slug')
New integration from plain authentication data
from snowflake.connector import connect
snowflake_connection = connect(
user="username",
password="password",
# You should use a yepcode env variable to don't store plain password
# See: https://docs.yepcode.io/docs/processes/team-variables
account="account",
database="database",
schema="schema",
)
Execute Statement
Execute statement
cursor = snowflake_connection.cursor()
cursor.execute("SELECT * FROM sales_stock WHERE COLOR_ID = 38379663 LIMIT 10;")
for item in cursor:
print(item)
Execute Statement with Parameters
Execute statement with parameters (literals)
cursor = snowflake_connection.cursor()
cursor.execute(
"SELECT * FROM sales_stock WHERE COLOR_ID = %s LIMIT 10;", (
"38379663"
)
)
for item in cursor:
print(item)
Execute Statement with Parameters
Execute statement with parameters (bind variables)
cursor = snowflake_connection.cursor()
cursor.execute(
"SELECT * FROM sales_stock WHERE COLOR_ID = %(color_id)s LIMIT 10", {
"color_id": 38379663,
}
)
for item in cursor:
print(item)
Insert Statement
Insert statement
cursor = snowflake_connection.cursor()
cursor.execute(
'INSERT INTO yourtable("Name","Number") VALUES(%s,%d)', (
'some-name',
4023
)
)
Select Statement
Select statement
cursor = snowflake_connection.cursor()
cursor.execute("SELECT * FROM sales_stock WHERE COLOR_ID = 38379663 LIMIT 10;")
for item in cursor:
print(item)
Destroy Connection
Terminating a connection
snowflake_connection.close()