Using User Modules
YepCode Modules allow you to define an isolated set of JavaScript or Python functions for reuse in any of your processes. These modules are designed to help share functions across processes, solve business logic problems, encapsulate access to services, and more.
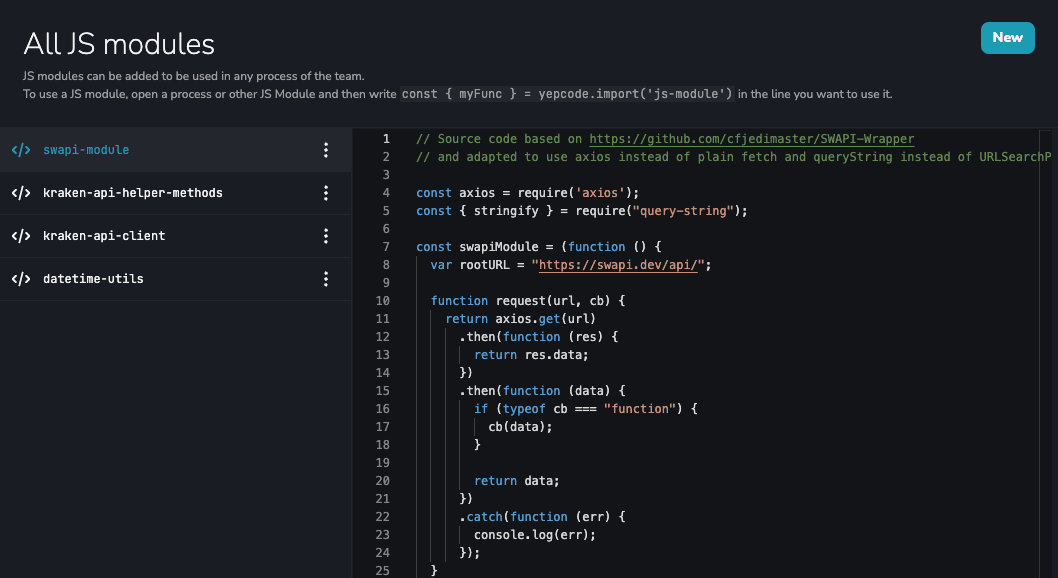
Modules function slightly differently in JavaScript and Python. Here's a guide for both:
JavaScript
Python
To use a module, use the custom import syntax in the process (or other module) where you want to utilize it.
const { myFunc } = yepcode.import("your-module");
Modules also support versioning and aliases, and if you want to import one specific module version or alias, just add a second parameter to the import sentence:
const { myFunc } = yepcode.import("your-module", "v1.0");
You can have as many modules as needed, and they work like any CommonJS module, exporting the functions you want to use from process source code.
For example, a JavaScript module exporting a function to say hello:
module.exports = () => console.log("Hello world!");
To use this library from a YepCode process named say_hello, the code would be:
const sayHello = yepcode.import("say_hello");
sayHello();
A module can also export several functions:
const sayHelloToMike = () => console.log("Hello Mike!");
const sayHelloToDavid = () => console.log("Hello David!");
module.exports = { sayHelloToMike, sayHelloToDavid };
To use these functions, read them from the returned object:
const sayHelloModule = yepcode.import("say_hello");
sayHelloModule.sayHelloToMike();
sayHelloModule.sayHelloToDavid();
To use a module, use the custom import syntax in the process (or other module) where you want to use it.
my_func = yepcode.import_module("your-module");
Modules also support versioning and aliases, and if you want to import one specific module version or alias, just add a second parameter to the import sentence:
my_func = yepcode.import_module("your-module", "v1.0");
You can have as many modules as needed, and they work like any Python module.
For example, a Python module exporting several functions:
def say_hello_to_mike():
print("Hello Mike!")
def say_hello_to_david():
print("Hello David!");
To use these functions, read them from the returned object:
say_hello_module = yepcode.import_module("say_hello");
say_hello_module.say_hello_to_mike();
say_hello_module.say_hello_to_david();
Writing Modules from Process Edition Screen
You can easily view and change modules from the process edition page by opening a component with this shortcut:
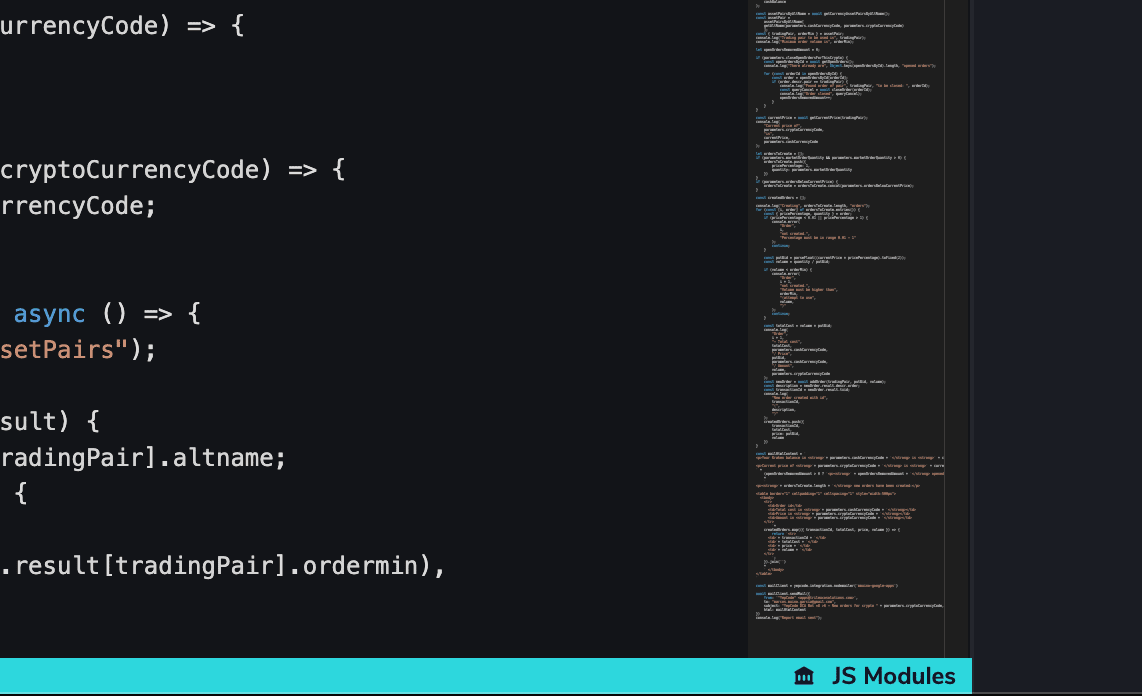
A modal window then shows you the defined modules, making it easy to modify or consult them.
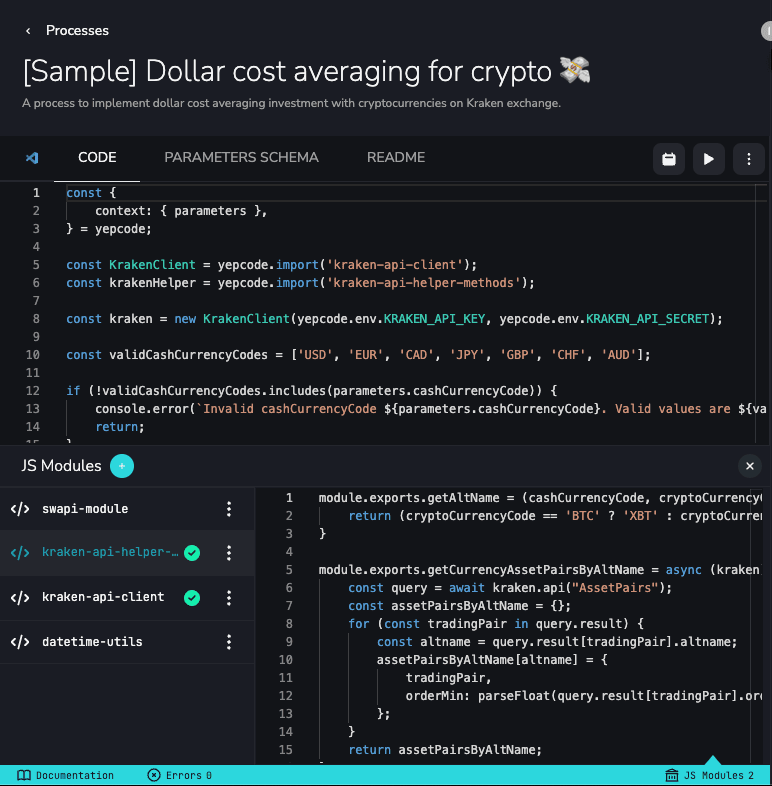